In Windows Forms, TextBox plays an important role. With TextBox, users can enter data in the application, which can be single or multi-line. TextBox is a class that defines the System.Windows.Forms namespace below. In C#, you can create a TextBox in two different ways:
1. Design time: This is the easiest way to create a TextBox, as shown in the following steps:
Step 1:
Create a Windows Form. As shown in the figure below:
Visual Studio > File > New > Project > WindowsFormApp

Step 2:
Drag and drop the text box control from the toolbox onto the Windows Forms. You can place the TextBox anywhere on Windows Forms as needed.
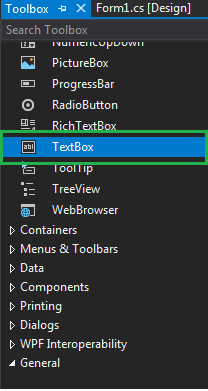
Step 3:
After dragging and dropping, you’ll go to the properties of the TextBox control to modify the TextBox design as needed.
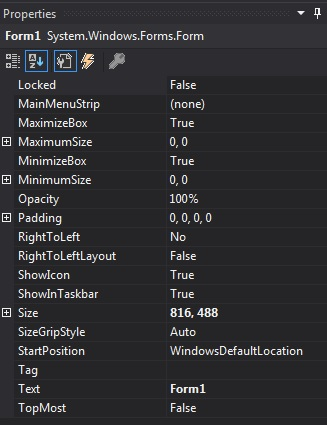
2. Runtime: It’s a bit trickier than the method above. In this approach, you can use the TextBox class to create your own text box.
Step 1: Create a text box using the TextBox() constructor provided by the TextBox class. create a text box TextBox Mytextbox = new TextBox();
Step 2: After creating the TextBox, set the properties of the TextBox provided by the TextBox class. Set the position of the Mytextbox text box. Location = new Point(187, 51); Set the background color of the text boxMytextbox.BackColor = Color.LightGray; set the text box Mytextbox.ForeColor = Color.DarkOliveGreen; foreground; Set the size of the text box Mytextbox.AutoSize = true; Set the name of the text box Mytextbox.Name=”text_box1″;
Step 3:
Finally add this text box control to use
Add() method
.
//Add this textbox to form
this.Controls.Add(Mytextbox);
Example:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace my {
public partial class Form1 : Form {
public Form1()
{
InitializeComponent();
}
private void Form1_Load( object sender, EventArgs e)
{
//Creating and setting the properties of Lable1
Label Mylablel = new Label();
Mylablel.Location = new Point(96, 54);
Mylablel.Text = "Enter Name" ;
Mylablel.AutoSize = true ;
Mylablel.BackColor = Color.LightGray;
//Add this label to form
this .Controls.Add(Mylablel);
//Creating and setting the properties of TextBox1
TextBox Mytextbox = new TextBox();
Mytextbox.Location = new Point(187, 51);
Mytextbox.BackColor = Color.LightGray;
Mytextbox.ForeColor = Color.DarkOliveGreen;
Mytextbox.AutoSize = true ;
Mytextbox.Name = "text_box1" ;
//Add this textbox to form
this .Controls.Add(Mytextbox);
}
}
}
The output is as follows:

Important properties of TextBox
AcceptsReturn | This property is used to set a value that is displayed in a multi-line TextBox control whether pressing the ENTER key in the control is to create a new line of text in the control or to activate the default button for a given form. |
AutoSize | This property is used to resize the TextBox based on the contents. |
BackColor | This property is used to set the background color of the text box. |
BorderStyle | This property is used to adjust the border type of the text box. |
CharacterCasing | This property is used to check if the TextBox control has modified the case of the characters when typing. |
Events | This property is used to provide a list of event handlers attached to this component. |
Font | This property is used to adjust the font of the text displayed by the text box control. |
ForeColor | This property is used to adjust the foreground color of the text box control. |
Location | This property is used to adjust the coordinates of the upper-left corner of a textbox control relative to the upper-left corner of its form. |
Margin | This property is used to set the margin between two textbox controls. |
MaxLength | This property sets the maximum number of characters that a user can type or paste into a text box control. |
Multiline | This property is used to set a value that shows whether this is a multi-line TextBox control. |
Name | This property is used to give the TextBox control a name. |
PasswordChar | This property is used to set the characters used to mask the password characters in a single-line TextBox control. |
ScrollBars | This property is used to set which scrollbars should appear in a multi-line TextBox control. |
Text | This property is used to set the text associated with this control. |
TextAlign | This property is used to adjust the alignment of the Chinese text of the TextBox control. |
TextLength | This property is used to get the length of the Chinese text of the TextBox control. |
UseSystemPasswordChar | This property is used to set a value that shows whether the text in the TextBox control should be displayed as the default password character. |
Visible | This property is used to obtain or set a value that determines whether the control and all of its children are displayed. |