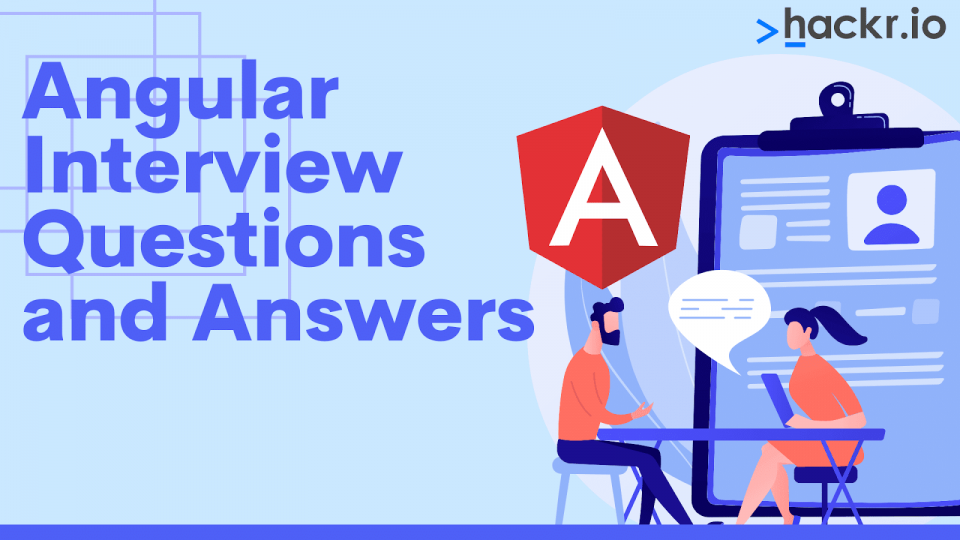
Ready to get your dream job as an Angular developer? Or, just planning to switch careers and become an Angular developer? In this article, we’ll look at some of the common Angular questions that you might ask in your Angular interview for your role as an Angular developer.
In addition to these concept-based questions, one (or more) coding tests are required. If you want to revise important Angular concepts before your interview, the full Angular course: udemy’s beginner to advanced course will prove to be very helpful. Apart from Angular questions, you will also be asked general interview questions. Here are 189 of the most popular programming problems and solutions to get you up and running quickly.
A collection of popular Angular interview questions and answers
So, are you ready to see how your preparations are going? Without further ado, we are here to show you some important Angular interview questions and answers that might be what you would expect in an interview. These questions also apply to your Angular 6 interview questions and Angular 7 interview questions.
Question: What is Angular?
A: Angular is an open-source web application framework based on TypeScript that is developed and maintained by Google. It provides a simple yet powerful way to build web-based front-end applications.
Angular integrates a range of features such as declarative templates, dependency injection, end-to-end tools, and more that facilitate web application development.
Question: Why was Angular introduced as a client-side framework?
Angular Interview Questions Explained: Traditionally, developers have used VanillaJS and jQuery to develop dynamic websites. As websites become more complex, adding features and functionality, it becomes difficult for developers to maintain the code. In addition, jQuery does not provide data processing facilities across views. As such, Angular aims to solve these problems and, as a result, make it easier for developers by dividing the code into smaller pieces of information (called components in Angular).
Client-side frameworks allow for the development of advanced web applications such as SPAs, which is a slower process if developed by VanillaJS.
What are the common Angular interview questions: Define ng-content directives?
A: Traditional HTML elements have some content between tags. For example:
<p>Put your paragraphs here</p>
Now consider the following example of having custom text between Angular tags:
<app-work>This won't work like HTML unless you use the ng-content directive</app-work>
However, doing so won’t work as well as it does for HTML elements. In order for it to work like the HTML example mentioned above, we need to use the ng-content directive. In addition, it helps in building reusable components.
Learn more about ng-content directives.
Question: Please explain the various features of Angular.
A: Angular has several features that make it an ideal front-end JavaScript framework. The most important of these are described below:
- Accessibility
Angular allows for the creation of accessible applications using ARIA-enabled components, built-in a11y testing infrastructure, and developer guides.
- Angular CLI
Angular provides support for command line interface tools. These tools can be used to add components, test, deploy instantly, and more.
- Animation support
Angular’s intuitive API allows for the creation of high-performance, complex animation timelines with very little code.
- Cross-platform app development
Angular can be used to build efficient and powerful desktop, native, and progressive web applications. Angular supports building native mobile apps using Cordova, Ionic, or NativeScript.
Angular allows you to create high-performance, offline, and zero-step installed progressive web applications using modern web platform capabilities. Popular JS frameworks can also be used to build desktop applications for Linux, macOS, and Windows.
- Code generation
Angular has the ability to convert templates into highly optimized code for a modern JavaScript virtual machine.
- Code splitting
With the new component router, Angular applications load quickly. The component router provides automatic code splitting so that only the code that is needed to render the view requested by the user is loaded.
- Synergies with popular code editors and IDEs
Angular provides code completion, on-the-fly errors, and more through popular source code editors and IDEs.
- template
Allows you to create UI views using simple yet powerful template syntax.
- Test
Angular allows you to use Karma to perform frequent unit tests. Angular allows faster scenario tests to be run in a stable manner.
Question: State some of Angular’s advantages over other frameworks.
Reply:
Out-of-the-box features: Several built-in features, such as routing, state management, rxjs libraries, and HTTP services, are out-of-the-box services provided by Angular. Therefore, there is no need to look for the above characteristics alone.
Declarative UI: Angular uses HTML to render an application’s UI because it’s a declarative language and easier to use than JavaScript.
Long-term Google support: Google plans to continue using Angular and further expand its ecosystem, as it already has long-term support for Angular.
Question: What is the difference between Angular and AngularJS?
Angular Interview Questions Analysis:
parameter | AngularJS | Angular |
architecture | The MVC or Model-View-Controller architecture facilitates the AngularJS framework, where the model contains the business logic, the controller processes the information, and finally, the view shows the information present in the model. | Angular replaces controllers with components with directives with predefined templates. |
language | AngularJS uses the JavaScript language, which is a dynamically typed language. | Angular uses the TypeScript language, a statically typed language, as well as a superset of JavaScript. Angular provides better performance when developing larger applications. |
Mobile support | Mobile phone support is not supported. | It is supported by all popular mobile browsers. |
structure | In the case of large applications, the process of maintaining code becomes tedious. | It’s easier to maintain code for large applications because it provides a better structure. |
Expression syntax | Developers need to remember the correct ng directive for binding events or properties. | Property bindings are done using the “[ ]” property, and event bindings are done using the “( )” property. |
routing | AngularJS uses $routeprovider.when() | Angular uses @RouteConfig{(…)} |
velocity | Due to bi-directional data binding, development effort and time are significantly reduced | Angular is faster due to the upgraded features. |
Dependency injection | AngularJS does not support DI. | Angular supports hierarchical dependency injection based on change detection of one-way trees. |
In the tank | There is no official support or update for AngularJS. | Angular actively supports rolling out updates from time to time. |
Question: What are lifecycle hooks in Angular? Explain some lifecycle hooks
A: The entire lifecycle of an Angular component from creation to destruction enters its lifecycle. Angular hooks provide a way to get into these stages and trigger changes at specific stages of the lifecycle.
ngOnChanges(): This method is called whenever one or more of the component’s input properties change. The hook receives a SimpleChanges object that contains the previous and current values of the property.
ngOnInit(): This hook is called once after the ngOnChanges hook.
It initializes the component and sets the input properties of the component.
ngDoCheck(): This is called after ngOnChanges and ngOnInit and is used to detect and handle changes that Angular can’t detect.
We can implement our change detection algorithm in this hook.
ngAfterContentInit(): It is called after the first ngDoCheck hook. This hook responds after the content is projected into the component.
ngAfterContentChecked(): It is called after ngAfterContentInit and each subsequent ngDoCheck. After inspecting the projected content, it responds.
ngAfterViewInit(): It responds after the component view or subcomponent view is initialized.
ngAfterViewChecked(): Called after ngAfterViewInit, responds after the component view or child component view has been checked.
ngOnDestroy(): It is called before Angular destroys the component. This hook can be used to clean up code and detach event handlers.
Question: Can we make an Angular application to render server-side?
A: Yes, we can use Angular Universal, which is a technology provided by Angular that enables server-side rendering of applications.
The benefits of using Angular Universal are:
- Better user experience: Allows users to see a view of the app immediately.
- Better SEO: Universal ensures that content is available on every search engine, resulting in better SEO.
- Faster loading: Rendered pages are faster to be available to browsers, so server-side applications load faster.
Question: Explaining Dependency Injection?
A: Dependency Injection is an application design pattern implemented by Angular that forms the core concept of Angular.
Let’s take a closer look. Dependencies in Angular are services that have functionality. The various components and directives in the application may require these features of the service. Angular provides a smooth mechanism through which these dependencies are injected into components and directives.
Problem: Describe the MVVM architecture.
A: The MVVM architecture eliminates tight coupling between each component. The MVVM architecture consists of three parts:
- model
- opinion
- View model
The architecture allows children to have a reference through observable objects rather than directly with their parents.
- Model: It represents the data and business logic of the application, or we can say that it contains the structure of an entity. It consists of business logic – local and remote data sources, model classes, repositories.
- View: A view is the visual layer of the application and therefore consists of UI code (in the component’s Angular-HTML template). It sends user actions to the ViewModel, but does not return a response directly. It must subscribe to the observables that the ViewModel exposes to it in order to get a response.
- ViewModel: It is the abstraction layer of the application that acts as a bridge between the View and the Model (business logic). It doesn’t know which View must use it because it doesn’t have a direct reference to the View. Views and ViewModels are associated with data binding, so any changes in the ViewModel in the View will record and change the data inside the Model. It interacts with the Model and exposes the observables that can be observed by the View.
Angular Interview Questions and Answers Collection: Demonstrate how to navigate between different routes in an Angular application.
A: The following code demonstrates how to navigate between different routes in an Angular app called “Some Search App”:
import from "@angular/router";
.
.
.
@Component({
selector: 'app-header',
template: `
<nav class="navbar navbar-light bg-faded">
<a class="navbar-brand" (click)="goHome()">Some Search App</a>
<ul class="nav navbar-nav">
<li class="nav-item">
<a class="nav-link" (click)="goHome()">Home</a>
</li>
<li class="nav-item">
<a class="nav-link" (click)="goSearch()">Search</a>
</li>
</ul>
</nav>
`
})
class HeaderComponent {
constructor(private router: Router) {}
goHome() {
this.router.navigate(['']);
}
goSearch() {
this.router.navigate(['search']);
}
}
Question: What is AOT (Ahead-of-Time) compilation? What are its advantages?
A: Angular applications are made up of components and templates that browsers can’t understand. As a result, every Angular application needs to be compiled before running in the browser. The Angular compiler takes the JS code, compiles it, and then generates some JS code. It’s called AOT compilation, and it only happens once at a time per user.
Angular provides two ways to compile:
JIT (Just-in-Time) Compilation: The application is compiled in the browser at runtime
AOT (Ahead-of-Time) Compilation: The application is compiled during the build.
Advantages of AOT compilation:
- Fast rendering: The browser loads the executable code and renders it as soon as the application compiles before it runs inside the browser.
- Fewer Ajax requests: The compiler sends external HTML and CSS files with the application, eliminating AJAX requests to these source files.
- Minimize errors: Errors are easy to detect and handle during the build phase.
- Better security: The AOT compiler adds HTML and templates to the JS file before running the application in the browser, so there are no additional HTML files to read, providing better security for the application.
What are the Common Angular Interview Questions: Can you explain the services in Angular?
A: A singleton object in Angular that is instantiated only once during the lifetime of an application is called a service. An Angular service contains a way to maintain data throughout the lifecycle of an application.
The primary purpose of Angular services is to organize and share business logic, models, or data and functionality with various components of an Angular application.
The Angular service provides functionality that can be invoked from any Angular component, such as a controller or directive.
Q: Discuss the pros and cons of using Angular?
A: Here are the various benefits of using Angular:
- Ability to add custom directives
- Exceptional community support
- Facilitate client and server communication
- With powerful features such as animations and event handlers
- Follow the MVC schema architecture
- Provides support for both static and Angular templates
- Bidirectional data binding is supported
- Support for dependency injection, RESTful services, and validation
The disadvantages of using Angular are listed below:
- Complex SPAs are inconvenient and laggy to use due to their size
- Dynamic apps don’t always perform well
- Learning Angular takes a lot of effort and time
Question: Name some of the distinguishing features of Angular 7.
A: Unlike previous versions of Angular, the 7th major release is split in @angular/core. This is done to reduce the same size. In general, not every module is what an Angular developer needs. So, in Angular 7, there will be no more than 418 modules per split of @angular/core.
In addition, Angular 7 introduces drag-and-drop and virtual scrolling. The latter allows loading and unloading elements from the DOM. For virtual scrolling, the latest version of Angular comes with the package. In addition, Angular 7 comes with a new and enhanced version of ng-compiler.
Question: What is string interpolation in Angular?
A: Also known as mustache syntax, string interpolation in Angular refers to a special type of syntax that uses template expressions to display component data. These template expressions are enclosed in double curly braces, i.e., {{ }}.
JavaScript expressions executed by Angular are added to the curly braces, and the corresponding output is embedded in the HTML code. Typically, these expressions are updated and registered like a watch as part of the digest cycle.
Problem: Explain Angular authentication and authorization.
Answer: The user login credentials are passed to the authentication API on the server. After server-side validation of the credentials, a JWT (JSON Web Token) is returned. JWTs have information or attributes about the current user. The user is then identified using the given JWT. This is called authentication.
After a successful login, different users have different access levels. While some people have access to everything, others may have access limited to certain resources. The access level is authorized.
Here is a detailed post about Angular 7 – JWT Authentication Examples and Tutorial: http://jasonwatmore.com/post/2018/11/16/angular-7-jwt-authentication-example-tutorial
Angular Interview Questions and Answers Collection: Can you explain the concept of scope hierarchy in Angular?
A: Angular organizes $scope objects into a hierarchy that is typically used by views. This is called a range hierarchy in Angular. It has a root scope that can further contain one or more scopes called subscopes.
In the scope hierarchy, each view has its own $scope. As a result, variables set by a view’s view controller will remain hidden from other view controllers. The following is a typical representation of a scope hierarchy:
- Root $scope
- $scope for Controller 1
- $scope for Controller 2
- …
- ..
- .
- $scope for Controller n
Question: How do I use the CLI to generate a class in Angular 7?
Answer:
ng generate class Dummy [options]
This will result in a class called Dummy.
Question: Explain what is the difference between Angular and backbone.js?
Angular Interview Questions Explained: Here are the various significant differences between Angular and Backbone.js
- Architecture
Backbone.js uses an MVP schema and doesn’t provide any data binding process. Instead, Angular works on an MVC architecture and leverages bidirectional data binding to drive application activity.
- Community support
Having Google support has greatly improved the community support that the Angular framework has received. In addition, a large amount of documentation is available. While Backbone.js has great community support, it only documents Underscore.js templates and not much else.
- Data binding
Angular uses a two-way data binding process, so it’s a bit complicated. Conversely, Backbone.js doesn’t have any data-binding process and therefore has a simple API.
- DOM
The main focus of Angular JS is on effective HTML and dynamic elements that mimic the underlying data, rebuild the DOM according to specified rules, and then process the updated data records.
Backbone.js follows a direct DOM manipulation approach to represent data and application schema changes.
- Performance
Thanks to its bi-directional data binding capabilities, Angular delivers impactful performance for both small and large projects.
In small datasets or small web pages, Backbone.js has a significant advantage over Angular in terms of performance. However, since there isn’t any data binding process, it’s not recommended for larger web pages or large datasets.
- Template making
Angular supports templating via dynamic HTML attributes. These are added to the documentation to develop easy-to-understand applications at the functional level. Unlike Angular, Backbone.js uses Underscore.js templates, which are not as functionally complete as Angular’s templates.
- Test Method:
The testing methods between Angular and Backbone.js vary widely, as the former is better suited for building large applications, while the latter is ideal for developing smaller web pages or applications.
For Angular, unit testing is preferred, and the testing process is smoother through the framework. In the case of Backbone.js, there is no data binding process, which allows for a fast testing experience for both individual pages and small applications.
- type
Angular is an open-source JS-based front-end web application framework that extends HTML with new attributes. Backbone.js, on the other hand, is a lightweight JavaScript library with a RESTful JSON interface and an MVP framework.
Q: How are Observables different from Promises?
A: As soon as a commitment is made, it will be implemented. However, this is not the case with observables because they are lazy. This means that nothing will happen until the subscription is made. A promise handles a single event, while an observable is a stream that allows multiple events to be delivered. Callbacks are made for each event in the observable.
Q: Can you explain the difference between Angular and AngularJS?
A: The various differences between Angular and AngularJS are illustrated below:
- Architecture – AngularJS supports the MVC design model. Angular relies on components and directives
- Dependency Injection (DI) – Angular supports hierarchical dependency injection and tree-based one-way change detection. AngularJS does not support DI
- Expression syntax – In AngularJS, images or properties and events require specific ng directives. Angular, on the other hand, uses () and [] to mask events and complete property bindings, respectively
- Mobile support – AngularJS doesn’t have mobile support, whereas Angular does
- Recommended language – JavaScript is the recommended language for AngularJS, and TypeScript is the recommended language for Angular
- Routing – For routing, AngularJS uses $routeprovider.when() while Angular uses @RouteConfig{(…)}
- Speed – Development effort and time is significantly reduced due to support for two-way data binding in AngularJS. Still, Angular is faster due to feature upgrades
- Structure – With a simplified structure, Angular makes the development and maintenance of large applications easier. In contrast, the structure of AngularJS is more difficult to manage
- Support – There is no official support or update for AngularJS. Instead, Angular actively supports rolling out updates from time to time
Question: Observe the image below:
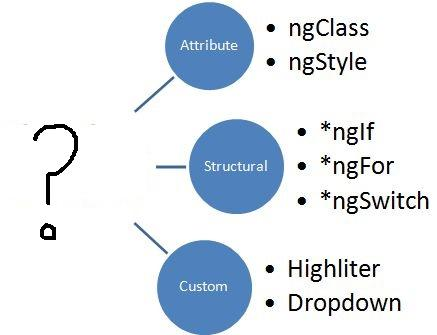
What to replace “? ”?
Answer: Instructions. This image represents the type of instruction in Angular; Properties, structure, and customization.
Question: Can you explain the concept of templates in Angular?
A: Templates in Angular are written in HTML and contain Angular-specific attributes and elements. Combined with information from the controller and model, the template is further rendered to suit the user’s dynamic view.
Question: Explain the difference between annotations and decorators in Angular?
A: In Angular, annotations are used to create annotation arrays. They’re just metadata sets of classes that use the Reflect Metadata library.
A decorator in Angular is a design pattern used to separate the decoration or modification of a class without changing the original source code.
Question: What are the directives in Angular?
A: Directives are one of Angular’s core features. They allow Angular developers to write new, application-specific HTML syntax. In fact, directives are functions that the Angular compiler executes when it finds them in the DOM. There are three types of directives:
- Attribute directives
- Component directives
- Structural directives
Question: What are the building blocks of Angular?
A: Angular applications basically have 9 building blocks. These are:
- Component – A component controls one or more views. Each view is a specific part of the screen. Every Angular application has at least one component, called the root component. It bootstraps in the main module, called the root module. Components contain the application logic defined in the class. This class is responsible for interacting with the view through the APIs of properties and methods.
- Data Binding – The mechanism by which parts of a template coordinate with parts of a component is called data binding. In order for Angular to know how to connect the two sides (the template and its components), binding tags were added to the template HTML.
- Dependency Injection (DI) – Angular uses DI to provide the required dependencies for new components. Typically, the dependencies required by a component are services. The component’s constructor argument tells the Angular component what service it needs. As a result, dependency injection provides a way to provide the fully formed dependencies required for a new instance of a class.
- Directives – The templates used through Angular are dynamic in nature. The directive is responsible for instructing Angular on how to convert the DOM when rendering the template. In fact, a component is a directive with a template. Other types of directives are attribute and struct directives.
- Metadata – In order for Angular to know what to do with a class, metadata is appended to the class. For this, decorators are used.
- Modules – Also known as NgModules, modules are organized chunks of code with a specific set of features. It has a specific application domain or workflow. Like components, any Angular application has at least one module. This is called the root module. Typically, an Angular application has multiple modules.
- Route-The Angular router is responsible for interpreting the browser URL as a navigation command to the view generated by the client. The router is bound to a link on the page to tell Angular to navigate the application view when the user clicks on it.
- Services – A very broad category, services can be anything from value and functionality to the functionality required for an Angular application. Technically, a service is a class with a clear purpose.
- Templates – The view of each component is associated with its companion template. A template in Angular is a form of HTML tag that lets Angular know how it renders components.
Q: Can you explain the difference between Angular and jQuery?
A: The biggest difference between Angular and jQuery is that the former is a JS front-end framework, while the latter is a JS library. Still, there are some similarities between the two, such as both having DOM manipulation and support for animation.
Nonetheless, the notable differences between Angular and jQuery are:
- Angular has two-way data binding, jQuery doesn’t
- Angular provides support for RESTful APIs, while jQuery does not
- jQuery doesn’t provide deep link routing, although Angular supports it
- There is no form validation in jQuery, it exists in Angular
What are the Common Angular Interview Questions: Can you explain the difference between Angular expressions and JavaScript expressions?
A: While both Angular and JavaScript expressions can contain literals, operators, and variables, there are some significant differences between the two. The important differences between Angular and JavaScript expressions are listed below:
- Angular expressions support filters, while JavaScript expressions don’t
- Angular expressions can be written inside HTML tags. Conversely, JavaScript expressions cannot be written in HTML tags
- While JavaScript expressions support conditions, exceptions, and loops, Angular expressions don’t
Question: Can you give us an overview of the Angular architecture?
A: You can draw something like this:
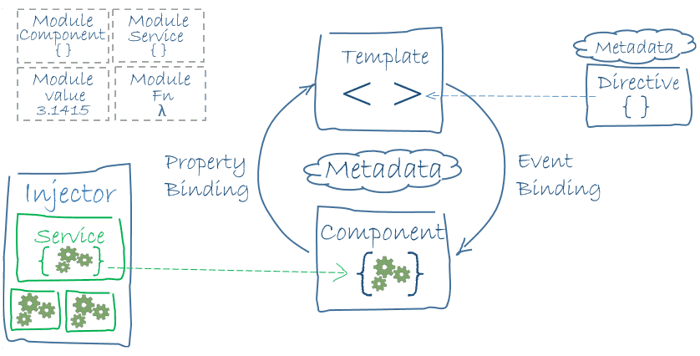
Here’s a closer look at Angular Architecture: https://angular.io/guide/architecture
Q: What is Angular material?
A: It’s a library of UI components. Angular Material helps create attractive, consistent, and fully functional web pages and web applications. It follows modern web design principles, including browser portability and elegant degradation.
Question: What is AOT (Ahead-of-Time) compilation?
A: Every Angular application is compiled internally. The Angular compiler takes the JS code, compiles it, and then generates some JS code. It only happens once per user at a time. It’s called AOT (Ahead-of-Time) compilation.
Question: What is data binding? How many ways can this be done?
A: In order to connect application data with the DOM (Data Object Model), data binding is used. It happens between templates (HTML) and components (TypeScript). There are 3 ways to implement data binding:
- Event binding – enables the application to respond to user input in the target environment
- Attribute Bindings – Allows values calculated from application data to be inserted into HTML
- Bi-directional binding – Changes made in the application state are automatically reflected in the view and vice versa. The ngModel directive is used to implement this type of data binding.
Question: What do the arrows in the image below represent?
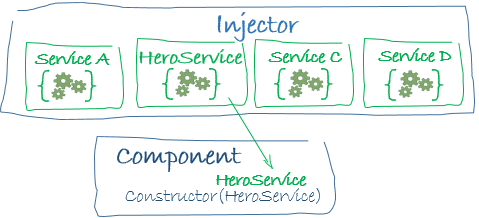
A: This stands for Dependency Injection or DI.
Question: Can you compare the service() and factory() functions?
A: For the business layer of an application, the service() function runs as a constructor. The function is called at runtime with the new keyword.
Although the factory() function works in much the same way as the service() function, the former is more flexible and powerful. In fact, the factory() function is a design pattern that helps create objects.
Question: Can you explain the summary loop in Angular?
A: The process of monitoring a watchlist to track changes in the values of the monitored variables is called a digest loop in Angular. Compare the previous and current versions of the range model values in each summary cycle.
Although the digest loop process is implicitly triggered, you can start it manually using the $apply() function.
Angular Interview Questions & Answers Collection: Can You Explain the Various Types of Filters in AngularJS?
A: In order to format the value of an expression so that it can be displayed to the user, AngularJS has filters. You can add these filters to a controller, directive, service, or template. AngularJS also supports the creation of custom filters.
Filters allow you to organize your data in such a way that it is displayed only when certain conditions are met. Using the pipeline | Add a filter to an expression feature. The various types of AngularJS filters are listed below:
- Currency – Format the number in a currency format
- Date – Format the data into a specific format
- filter – Selects a subset of items from the array
- json – Format the object as a JSON string
- limitTo – Limits an array or string to a specified number of characters or elements
- Lowercase – Format the string in lowercase
- number – Format the number as a string
- orderBy – Sort an array by expression
Q: What’s new in Angular 6?
A: Here are some of the new aspects introduced in Angular 6:
- Angular Elements – It allows Angular components to be converted into web components and embedded in some non-Angular applications
- Tree Shakeable Provider – Angular 6 introduces a new way to register a provider directly in the @Injectable() decorator. It is achieved by using the providedIn property
- RxJS 6 – Angular 6 uses RxJS 6 internally
- i18n (Internationalization) – No need to build an application once for each locale, any Angular application can have a “runtime i18n”
Question: What is ngOnInit()? How to define it?
Angular Interview Question Explained: ngOnInit() is a lifecycle hook that is called after Angular has initialized all the data-bound properties of the directive. It is defined as:
Interface OnInit {
ngOnInit() : void
}
Question: What is a SPA (Single Page Application) in Angular? How does SPA technology compare with traditional web technology?
A: In SPA technology, while the URL is constantly changing, only one page, the index.HTML, is maintained. Unlike traditional web technologies, SPA technology is also faster and easier to develop.
In traditional web technologies, whenever a client requests a web page, the server sends a resource. However, when the client requests another page again, the server responds again to the resource that sent the request. The problem with this technology is that it takes a lot of time.
Question: What is the code for creating decorators?
A: We created a decorator called Dummy:
function Dummy(target) {
dummy.log('This decorator is Dummy', target);
}
Question: What is the process of converting TypeScript code to JavaScript code?
A: It’s called translation. Although TypeScript is used to write code in an Angular application, it is internally converted to the equivalent JavaScript.
Question: What is ViewEncapsulation and how many ways are there to do it in Angular?
A: In simple terms, ViewEncapsulation determines whether the styles defined in a particular component affect the entire application. Angular supports 3 types of ViewEncapsulation:
- Emulated—Styles used in other HTML are propagated to the component
- Native – Styles used in other HTML are not propagated to the component
- None – The styles defined in the component are visible to all components of the application
Question: Why is TypeScript prioritized over JavaScript in Angular?
A: TypeScript is a superset of Javascript because it is Javascript + Types or extra features such as type conversion of variables, annotations, variable ranges, and so on. TypeScript is designed to overcome Javascript shortcomings such as type conversion of variables, classes, decorators, variable ranges, and more. In addition, Typescript is pure object-oriented programming, which provides a “compiler” that can be converted into code equivalent to Javascript.
Question: Discuss the lifecycle of directives and components designed in Angular JS, specifically the newly introduced 6.0 release?
Reply:
The components and directives of Angular JS follow the following typical lifecycle.
- nhOnInit
- ngDoCheck
- ngOnDestroy
- Constructor
- ngOnChanges
- ngAfterContentInit (for components only)
- ngAfterContentChecked (for components only)
- ngAfterViewInit (for components only)
- ngAfterViewChecked (for components only)
Problem: Writing out Angular 6 features on Angular 5.
A: It has the following characteristics:
1. Added NG update
The CLI command updates the angular project dependencies to the latest version. ng update is a normal package manager tool that is used to identify and update in a normal package manager tool to identify and update other dependencies.
2. Use RxJS6
This is a third-party library (RxJS) that introduces two important changes compared to RxJS5.
- Introducing a new internal package structure.
- Operator concept
To update RxJS6, run the following command:
npm install --save rxjs@6
To update an existing Angular project, run the following command:
npm install --save rxjs-compat
3. <ng-template>
Angular 6 uses <ng-template> instead of <template>
4. Changes in Service Levels
If, in earlier versions, the user wanted to serve the entire application, the user would need to add it to the provider in the AppModule, but not in the case of Angular 6.
5. Rename the operator
Angular 6 renames the following operators:
- do() => tap()
- catch() => catchError()
- finally() => finalize()
- switch()=>switchAll()
- throw() => throwError
Question: Why was Angular introduced as a client-side framework?
A: Traditionally, developers have used VanillaJS and jQuery to develop dynamic websites. As websites become more complex, adding features and functionality, it becomes difficult for developers to maintain the code. In addition, jQuery does not provide data processing facilities across views. As such, Angular aims to solve these problems and, as a result, make it easier for developers by dividing the code into smaller pieces of information (called components in Angular). Client-side frameworks allow for the development of advanced web applications such as SPAs, which is a slower process if developed by VanillaJS.
Question: State some of Angular’s advantages over other frameworks.
Reply:
- Out-of-the-box features: Several built-in features, such as routing, state management, rxjs libraries, and HTTP services, are out-of-the-box services provided by Angular. Therefore, there is no need to look for the above characteristics alone.
- Declarative UI: Angular uses HTML to render an application’s UI because it’s a declarative language and easier to use than JavaScript.
- Long-term Google support: Google plans to continue using Angular and further expand its ecosystem, as it already has long-term support for Angular.
Question: What is the difference between Angular and AngularJS?
Reply:
parameter | AngularJS | Angular |
architecture | The MVC or Model-View-Controller architecture facilitates the AngularJS framework, where the model contains the business logic, the controller processes the information, and finally, the view shows the information present in the model. | Angular replaces controllers with components with directives with predefined templates. |
language | AngularJS uses the JavaScript language, which is a dynamically typed language. | Angular uses the TypeScript language, a statically typed language, as well as a superset of JavaScript. Angular provides better performance when developing larger applications. |
Mobile support | Mobile phone support is not supported. | It is supported by all popular mobile browsers. |
structure | In the case of large applications, the process of maintaining code becomes tedious. | It’s easier to maintain code for large applications because it provides a better structure. |
Expression syntax | Developers need to remember the correct ng directive for binding events or properties. | Property bindings are done using the “[ ]” property, and event bindings are done using the “( )” property. |
routing | AngularJS uses $routeprovider.when() | Angular uses @RouteConfig{(…)} |
velocity | Due to bi-directional data binding, development effort and time are significantly reduced | Angular is faster due to the upgraded features. |
Dependency injection | AngularJS does not support DI. | Angular supports hierarchical dependency injection based on change detection of one-way trees. |
In the tank | There is no official support or update for AngularJS. | Angular actively supports rolling out updates from time to time. |
What are the Common Angular Interview Questions: What is AOT (Ahead-of-Time) Compilation? What are its advantages?
A: Angular applications are made up of components and templates that browsers can’t understand. As a result, every Angular application needs to be compiled before running in the browser. The Angular compiler takes the JS code, compiles it, and then generates some JS code. It’s called AOT compilation, and it only happens once at a time per user. Angular provides two ways to compile:
- JIT (Just-in-Time) Compilation: The application is compiled in the browser at runtime
- AOT (Ahead-of-Time) Compilation: The application is compiled during the build.
Advantages of AOT compilation:
- Fast rendering: The browser loads the executable code and renders it as soon as the application compiles before it runs inside the browser.
- Fewer Ajax requests: The compiler sends external HTML and CSS files with the application, eliminating AJAX requests to these source files.
- Minimize errors: Errors are easy to detect and handle during the build phase.
- Better security: The AOT compiler adds HTML and templates to the JS file before running the application in the browser, so there are no additional HTML files to read, providing better security for the application.
Question: What are lifecycle hooks in Angular? Explain some lifecycle hooks.
A: The entire lifecycle of an Angular component from creation to destruction enters its lifecycle. Angular hooks provide a way to get into these stages and trigger changes at specific stages of the lifecycle.
- ngOnChanges(): This method is called whenever one or more of the component’s input properties change. The hook receives a SimpleChanges object that contains the previous and current values of the property.
- ngOnInit(): This hook is called once after the ngOnChanges hook.
- It initializes the component and sets the input properties of the component.
- ngDoCheck(): This is called after ngOnChanges and ngOnInit and is used to detect and handle changes that Angular can’t detect.
- We can implement our change detection algorithm in this hook.
- ngAfterContentInit(): It is called after the first ngDoCheck hook. This hook responds after the content is projected into the component.
- ngAfterContentChecked(): It is called after ngAfterContentInit and each subsequent ngDoCheck. After inspecting the projected content, it responds.
- ngAfterViewInit(): It responds after the component view or subcomponent view is initialized.
- ngAfterViewChecked(): Called after ngAfterViewInit, responds after the component view or child component view has been checked.
- ngOnDestroy(): It is called before Angular destroys the component. This hook can be used to clean up code and detach event handlers.
Question: Can we make an Angular application to render server-side?
Angular Interview Question Explained: Yes, we can use Angular Universal, which is a technology provided by Angular that is capable of rendering applications on the server side. The benefits of using Angular Universal are:
- Better user experience: Allows users to see a view of the app immediately.
- Better SEO: Universal ensures that content is available on every search engine, resulting in better SEO.
- Faster loading: Rendered pages are faster to be available to browsers, so server-side applications load faster.
Question: Explaining Dependency Injection?
A: Dependency injection is an application design pattern implemented by Angular that forms the core concept of Angular. Let’s take a closer look. Dependencies in Angular are services that have functionality. The various components and directives in the application may require these features of the service. Angular provides a smooth mechanism through which these dependencies are injected into components and directives.
Problem: Describe the MVVM architecture.
A: The MVVM architecture eliminates tight coupling between each component. The MVVM architecture consists of three parts:
- Model
- View
- ViewModel
The architecture allows children to have a reference through observable objects rather than directly with their parents.
- Model: It represents the data and business logic of the application, or we can say that it contains the structure of an entity. It consists of business logic – local and remote data sources, model classes, repositories.
- View: The view is the visual layer of the application and therefore consists of UI code (in the component’s Angular-HTML template). It sends user actions to the ViewModel, but does not return a response directly. It must subscribe to the observables that the ViewModel exposes to it in order to get a response.
- ViewModel: It is the abstraction layer of the application that acts as a bridge between the View and the Model (business logic). It doesn’t know which View must use it because it doesn’t have a direct reference to the View. Views and ViewModels are associated with data binding, so any changes in the ViewModel in the View will record and change the data inside the Model. It interacts with the Model and exposes the observables that can be observed by the View.
Question: Are these questions relevant to Angular 6 interview questions?
Answer: Yes, they can be considered as Angular 6 interview questions.
Angular Interview Questions and Answers Collection Conclusion
When it comes to interviewing with Anterview, you need to be confident. Also, try to avoid nonsense just in case you get asked a question that you don’t know about. A simple one is better than giving some random non-answer and adding unnecessary details. Frank and honest candidates are preferred over candidates who pretend to know everything! Also, don’t reveal your personal details until you are asked to do so. The interviewer wants to know more about you being a techie. So, all the best! Please tell us about the Angular questions you had in your interview that were not covered here so that we can add them here for the benefit of the Angular community.
Are these questions also relevant to Angular JS interview questions? Yes, these questions are helpful when preparing for an AngularJS interview. Angular is a top-level programming language and is used by most web developers.