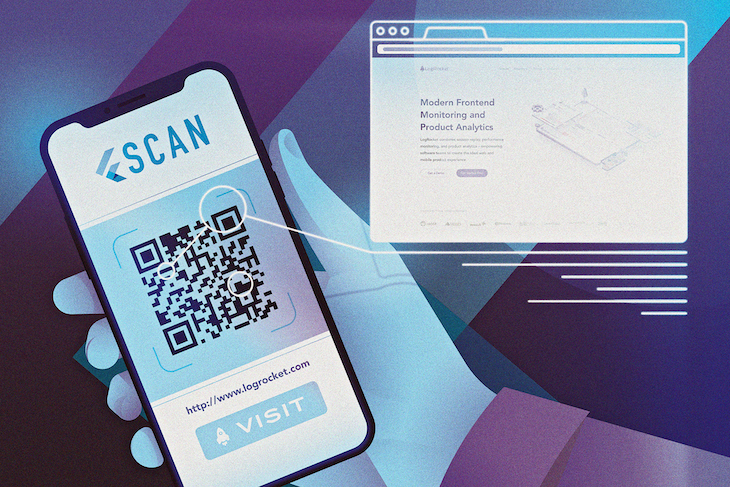
Flutter creates an instance of a barcode scanner
If you want people to use your app to identify data quickly and visually, you can’t use barcodes and QR codes. They have been around for a long time, optically identifying fragments of data without errors or misunderstandings.
Today, barcodes still have many uses. One of the more common uses we’ve seen recently is in restaurants, where customers can scan a QR code to order a specific item from a menu.
How to create a Flutter barcode scanner? In this article, this is exactly what we are going to show how barcodes and QR codes work in mobile apps: we are going to create a simple application called Scantastic that looks like this:
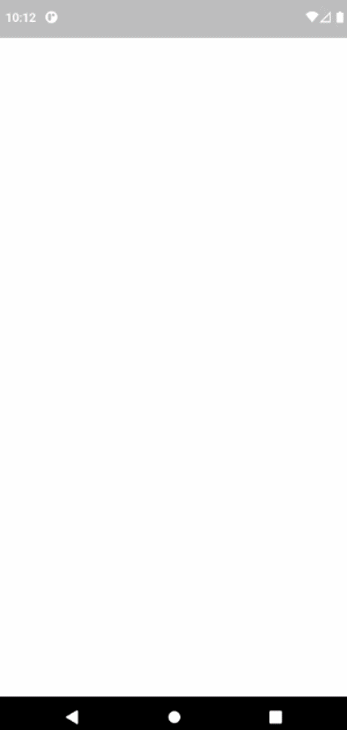
The app will let users scan a QR code and then identify it as a food item. How does Flutter barcode scanner? To create it, we must complete the following steps:
- Generate a QR code using our menu option
- Add a dependency
qr_code_scanner
that will allow us to scan QR codes and barcodes from our app - Add menu options to our app and react when these items are detected
- Configure Android Emulator to display QR codes in a simulated environment for testing
Get ready? Let’s get started.
Flutter Create Barcode Scanner Instance: Create a Flutter Project
The first thing we need to do is create our Flutter project, which we can do by typing in the command line.flutter create scantastic
Once the command is complete, we can add it to our project, which we can do by writing the following code to the command line:qr_code_scanner
flutter pub add qr_code_scanner
This increases the reliance on QR code scanning packages. Because we are going to use the camera on the user’s phone to carry out our task, we will have to make some settings to do the job.
Android platform configuration
In general, Flutter is broadly compatible with any version of Android, even very old ones. However, since the package uses features that are only available in Android KitHat, we have to adjust some configuration settings to influence this change.qr_code_scanner
To use this plugin, make sure you have the latest Gradle, Kotlin, and Kotlin Gradle plugins. To do this, follow the integration process outlined in the qr_code_scanner
repository.
Here we have to simply adjust the versions of Kotlin and Gradle used by our app and set the minimum SDK version of our app to . This simply means that the app can only run on Android KitKat and above.20
iOS platform configuration
How does Flutter barcode scanner? Because we are accessing the phone’s camera, the Apple App Store will see that we are making a request to access the camera and wonder why we are making that request.
We can configure iOS for the plugin by adding the following to the file:Info.plist
<key>io.flutter.embedded_views_preview</key>
<true/>
<key>NSCameraUsageDescription</key>
<string>This app needs camera access to scan QR codes</string>
Now, when users try to scan a QR code in the app with their camera, they will see a warning for them to accept or reject the app for using their camera.
Create our sample Flutter app
How to create a Flutter barcode scanner? Once our platform is configured, we can now create our QR code Flutter app. For ease of use, I split the project into several different files to avoid confusion. The layout of the project looks like this:
- libmain.dart (contains the start screen of our app)
- libhomehome.dart (including QR code scanning screen)
- libhomeorder.dart (screen that loads when the code is recognized)
- qrcodes (a directory containing a sample QR code to be used)
Since this article focuses on the process of scanning QR codes, we will spend most of our time looking at the files.home.dart
Create a list of recognizable items
Flutter creates a barcode scanner instance: The first thing we’re going to do is create an item that our scanners are supposed to recognize. We have to do this because our barcode scanner may be able to recognize any barcode, and we want to limit it to only reacting to a predefined list of items.List
We also want to associate a specific icon with each item so that the user is shown a visual representation of the item they will be ordering.
Our AN class looks like this:ExpectedScanResult
class ExpectedScanResult {
final String type;
final IconData icon;
ExpectedScanResult(this.type, this.icon);
}
We can then initialize the following code on our line 15:home.dart
final recognisedCodes = <ExpectedScanResult>[
ExpectedScanResult('cake', Icons.cake),
ExpectedScanResult('cocktail', Icons.local_drink_outlined),
ExpectedScanResult('coffee', Icons.coffee),
ExpectedScanResult('burger', Icons.fastfood_rounded),
];
This adds a list of items we accept at the time of scanning, including relevant icons.
Set the result
Barcode
The next thing we have to do is set our results and ours.Barcode
QRViewController
To do this, we’ll add these variables, but make them null by adding a suffix to them. We do this because we won’t know what our barcode result will be until the user scans the barcode, and we can only access ours after the QR code scanner is initialized:?
QRViewController
Barcode? result;
QRViewController? controller;
Configure the widget
QRView
How Flutter Barcode Scanner: Now, we can configure our widgets in our widget tree. In the sample app, we put the QR scanner in a widget so that we can tell the user what to scan when the QR code scanner starts.QRView
Stack
Let’s configure it like this: QRView
QRView(
cameraFacing: CameraFacing.back, // Use the rear camera
key: qrKey, // The global key for the scanner
onQRViewCreated: _onQRViewCreated, // Function to call after the QR View is created
overlay: QrScannerOverlayShape( // Configure the overlay to look nice
borderRadius: 10,
borderWidth: 5,
borderColor: Colors.white,
),
),
In the function, we pull in the list of foods we want to see. We can then subscribe to the events received from the QR code scanner._onQRViewCreated
Flutter creates a barcode scanner instance: if the value we’re scanning is in a list we accept, we’ll take the user to another page where they can confirm their order like this:
void _onQRViewCreated(QRViewController controller) {
this.controller = controller;
// Retrieve the list of expected values
final expectedCodes = recognisedCodes.map((e) => e.type);
// Subscribe to the incoming events from our QR Code Scanner
controller.scannedDataStream.listen((scanData) {
// If the scanned code matches any of the items in our list...
if (expectedCodes.any((element) => scanData.code == element)) {
// ... then we open the page confirming the order with our user
Navigator.of(context).push(
MaterialPageRoute(
builder: (context) => OrderPage(
// Pass in the recognised item to the Order Page
item: recognisedCodes.firstWhere((element) => element.type == scanData.code),
),
),
);
}
});
}
With this, we have laid the groundwork for a QR code scanner in our app. However, we now have a small problem: if we want to scan a piece of physical paper with a QR code, it will be a pain to deploy this app onto a physical device for debugging.
Luckily, the Android Emulator comes with its own virtual environment where we can take pictures. It also allows us to set up an image in the environment that we can use to test our QR code scans. Let’s see how to do it.
Configure the Android emulator for testing
How to create a Flutter barcode scanner? Once our code is ready to run or we have cloned the sample app, we can launch the Android Emulator. Depending on whether you’re using Visual Studio Code or Android Studio, how you do it will be different.
Once the Android Emulator is launched, open the camera settings to set up the image. First, click on the three dots at the bottom of the menu, then click on Camera and then add Image, as shown in the image below:
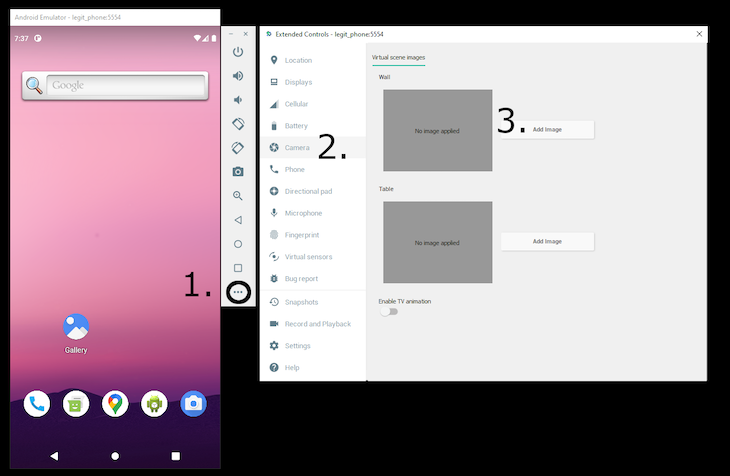
Flutter how barcode scanner
Next, select one of the images in the catalog. This allows the code to appear in the virtual camera environment.qrcodes
If you now open the camera in the emulator and hold down the left ALT key, use W to walk forward, and the mouse looks around to “walk over” to the location where the QR code is displayed, as shown below:
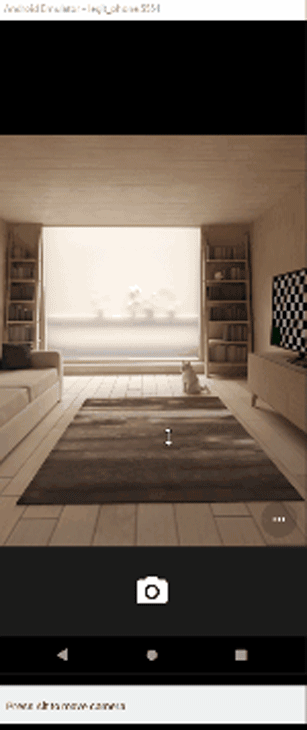
Flutter creates an instance of a barcode scanner
I’ll admit it’s complicated, placing the QR code on a wall in a virtual environment; But it’s still better than printing a piece of physical paper with a QR code and scanning it.
And, if you do the same in our sample app, the QR code will be recognized, and the user will see the option to order the item (as shown in the opening video).
Summary
How to create a Flutter barcode scanner? QR codes and barcodes are powerful tools that can be used to quickly identify data optically. In this article, we learned how to use the Flutter package to recognize these codes and work with them.
We also configured an emulator to display one of these codes so that we could use physical paper with the codes, speeding up our workflow.
Happy scanning!