Python OpenCV detects contours in an image: Contours are closed curves that connect all contiguous points of a certain color or intensity, and they represent the shape of the objects found in the image. Contour detection is a useful technique for shape analysis and object detection and recognition.
In the previous tutorial we talked about edge detection with Canny’s algorithm, and we’ve seen how to implement it in OpenCV, you might ask, what’s the difference between edge detection and contour detection?
How does OpenCV detect image contours? When we perform edge detection, we find points where the color intensity changes significantly, and then we simply turn on those pixels. However, an outline is an abstract collection of points and line segments that correspond to the shape of an object in an image. Thus, we can manipulate contours in our program, such as counting the number of contours, using them to classify the shape of objects, cropping objects from images (image segmentation), and much more.
Contour detection is not the only algorithm for image segmentation, there are many others, such as the current state-of-the-art semantic segmentation, Hough transform, and K-Means segmentation.
For better accuracy, here’s the entire process we follow to successfully detect contours in an image:
- To convert an image to a binary image, it is common practice to change the input image to a binary image (which should be the result of a threshold image or edge detection).
- Use the findContours() OpenCV function to find the outline.
- These outlines are drawn and the image is displayed.
Related: How to apply HOG feature extraction in Python.
OpenCV Detection Image Contour Example – Okay, let’s get started. First, let’s install the dependencies for this tutorial:
pip3 install matplotlib opencv-python
Import the necessary modules:
import cv2
import matplotlib.pyplot as plt
Python OpenCV detects contours in an image – we’ll use this image in this tutorial:
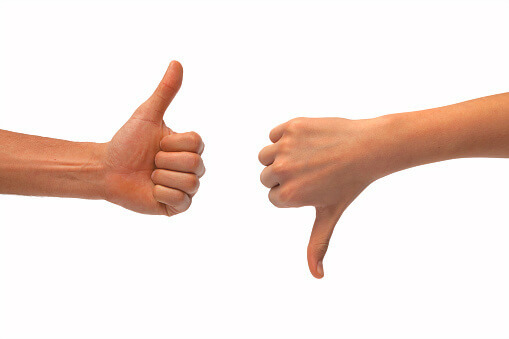
Let’s load it:
# read the image
image = cv2.imread("thumbs_up_down.jpg")
Convert it to RGB and then grayscale:
# convert to RGB
image = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
# convert to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_RGB2GRAY)
How does OpenCV detect image contours? As mentioned earlier in this tutorial, we need to create a binary image, which means that every pixel of the image is black or white. This is necessary in OpenCV, finding the outline is like finding a white object from a black background, the object to be found should be white, and the background should be black.
# create a binary thresholded image
_, binary = cv2.threshold(gray, 225, 255, cv2.THRESH_BINARY_INV)
# show it
plt.imshow(binary, cmap="gray")
plt.show()
The above code builds by disabling (set to binary image 0), which has a pixel 225 with less than one value, and on (set to 255), which has a pixel 225 with a value greater than one, here is the output image:

Python OpenCV detects contours in images – now this is easy for OpenCV to detect contours:
# find the contours from the thresholded image
contours, hierarchy = cv2.findContours(binary, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# draw all contours
image = cv2.drawContours(image, contours, -1, (0, 255, 0), 2)
OpenCV detects image outline example: The above code finds the outlines in the binary image and plots them onto the image with a thick green line, let’s show:
# show the image with the drawn contours
plt.imshow(image)
plt.show()
Output Image:

To get good results on different real-world images, you need to adjust thresholds or perform edge detection. For example, for a pancake image, I lowered the threshold to 127 and the result is as follows:

Ok, that’s what this tutorial is about, if you want to test it on a live camera, go to this link.