OpenCV (Open Source Computer Vision) is a computer vision library that contains various functions for performing operations on images or videos. The OpenCV library can be used to perform several operations on video.
Let’s see how to play a video using OpenCV Python.
In order to capture a video, we need to create a VideoCapture object. The video capture has the name of the device index or video file. The device index is simply a number that specifies which camera. If we pass 0, then the first camera is 0, the second camera is 1, and so on. We capture the video frame by frame.
Grammar:
cv2.VideoCapture(0): Means first camera or webcam.
cv2.VideoCapture(1): Means second camera or webcam.
cv2.VideoCapture("file name.mp4"): Means video file
Here’s the implementation:
# importing libraries
import cv2
import numpy as np
# Create a VideoCapture object and read from input file
cap = cv2.VideoCapture( 'tree.mp4' )
# Check if camera opened successfully
if (cap.isOpened() = = False ):
print ( "Error opening video file" )
# Read until video is completed
while (cap.isOpened()):
# Capture frame-by-frame
ret, frame = cap.read()
if ret = = True :
# Display the resulting frame
cv2.imshow( 'Frame' , frame)
# Press Q on keyboard to exit
if cv2.waitKey( 25 ) & 0xFF = = ord ( 'q' ):
break
# Break the loop
else :
break
# When everything done, release
# the video capture object
cap.release()
# Closes all the frames
cv2.destroyAllWindows()
Note: The video file should be in the same directory as the directory where the program is executed.
Video Sample Frames:
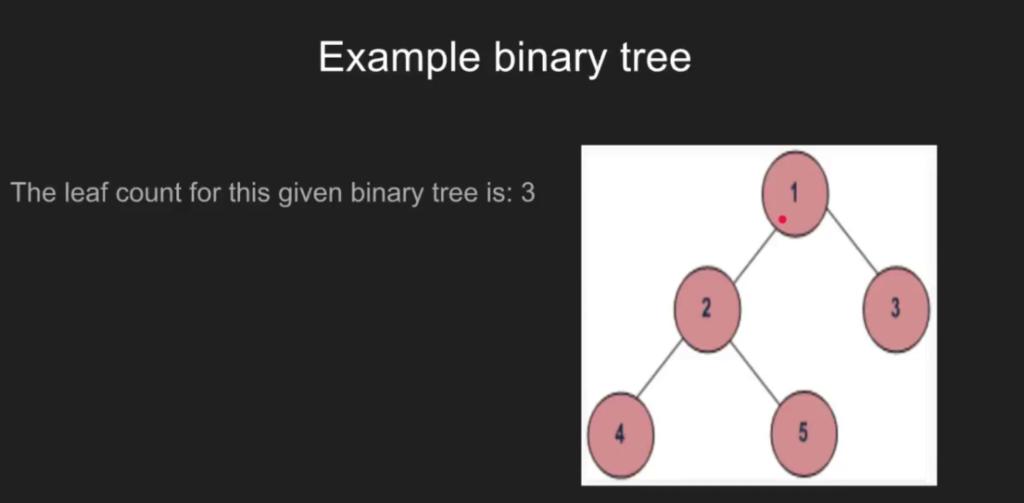
Related Article: How to Play a Video in Reverse Mode.
First, your interview preparation enhances your data structure concepts with the Python DS course.