How does Python change the color of text? This article walks you through how to use the colorama library to print colored text with different colors such as red, green, and blue in the background and foreground and brightness in Python.
How do I change the text color in Python? Printing to the console in different colors is very convenient and useful, from building beautiful scan scripts to distinguishing between different types of log messages in the program (debug, informational, critical, etc.). In this tutorial, you’ll learn how to print colored text in Python using the colorama
library.
Python change text color code example intro: we’ll use, let’s install it first:colorama
$ pip install colorama
Next, open a new Python file and write the following:
from colorama import init, Fore, Back, Style
# essential for Windows environment
init()
# all available foreground colors
FORES = [ Fore.BLACK, Fore.RED, Fore.GREEN, Fore.YELLOW, Fore.BLUE, Fore.MAGENTA, Fore.CYAN, Fore.WHITE ]
# all available background colors
BACKS = [ Back.BLACK, Back.RED, Back.GREEN, Back.YELLOW, Back.BLUE, Back.MAGENTA, Back.CYAN, Back.WHITE ]
# brightness values
BRIGHTNESS = [ Style.DIM, Style.NORMAL, Style.BRIGHT ]
First, we call the function, which is necessary for proper functioning in the Windows environment, it doesn’t work on other platforms, so you can remove it.init()
colorama
Second, we defined all the available foreground colors in the list, the background colors in the list, and we also defined the list for the different brightness settings.FORES
BACKS
BRIGHTNESS
Next, let’s create a function that wraps around a regular Python function, but with the ability to set color and brightness:print()
def print_with_color(s, color=Fore.WHITE, brightness=Style.NORMAL, **kwargs):
"""Utility function wrapping the regular `print()` function
but with colors and brightness"""
print(f"{brightness}{color}{s}{Style.RESET_ALL}", **kwargs)
We simply use inside, but precede the text with the brightness and color code, and append at the end to reset to the default color and brightness every time we use the function.print()
Style.RESET_ALL
We also pass so we can use the keyword arguments of other functions, such as and.**kwargs
print()
end
sep
How does Python change the color of text? Now that we have our function, let’s use all the foreground colors and print the same text with different colors and different brightness:
# printing all available foreground colors with different brightness
for fore in FORES:
for brightness in BRIGHTNESS:
print_with_color("Hello world!", color=fore, brightness=brightness)
This will look like the following image:
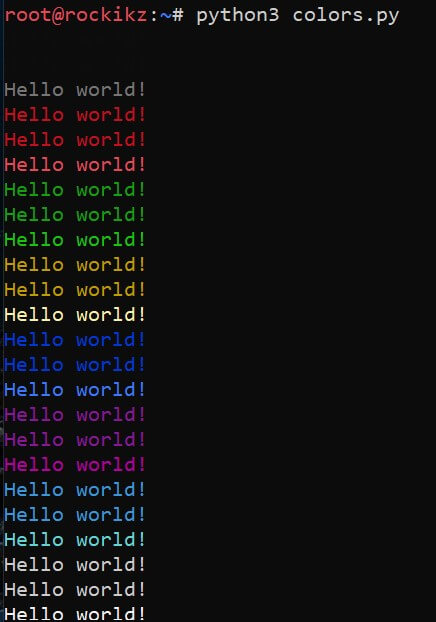
Black is not shown because the background color of the terminal is also black, and there are different background colors here:
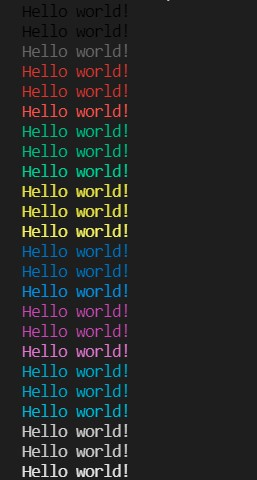
Python change text color code example – now let’s use the background color:
# printing all available foreground and background colors with different brightness
for fore in FORES:
for back in BACKS:
for brightness in BRIGHTNESS:
print_with_color("A", color=back+fore, brightness=brightness, end=' ')
print()
You can change both the background and the foreground color, which is why we iterated over the foreground color as follows:

Conclusion
How do I change the text color in Python? That’s it! Now you know all the foreground and background colors and brightness values available in the Python library. I hope this helps you get up to speed quickly and copy the code for your project.