Using the XLRD module, information can be retrieved from spreadsheets. For example, you can read, write, or modify data in Python. In addition, users may have to go through various worksheets and retrieve data based on certain criteria or modify certain rows and columns and do a lot of work.
The RD module is used to extract data from spreadsheets.
Command to install the xlrd module:
pip install xlrd
Input file:
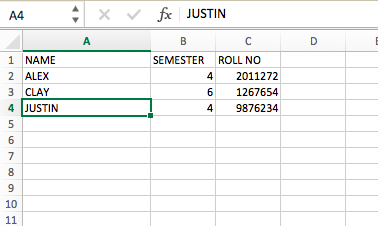
Code 1: Extract specific cells
Python3
# Reading an excel file using Python
import xlrd
# Give the location of the file
loc = ( "path of file" )
# To open Workbook
wb = xlrd.open_workbook(loc)
sheet = wb.sheet_by_index( 0 )
# For row 0 and column 0
print (sheet.cell_value( 0 , 0 ))
Output:
'NAME'
Code 2: Extract the number of rows
Python3
# Program to extract number
# of rows using Python
import xlrd
# Give the location of the file
loc = ( "path of file" )
wb = xlrd.open_workbook(loc)
sheet = wb.sheet_by_index( 0 )
sheet.cell_value( 0 , 0 )
# Extracting number of rows
print (sheet.nrows)
Output:
4
Code 3: Extract the number of columns
Python3
# Program to extract number of
# columns in Python
import xlrd
loc = ( "path of file" )
wb = xlrd.open_workbook(loc)
sheet = wb.sheet_by_index( 0 )
# For row 0 and column 0
sheet.cell_value( 0 , 0 )
# Extracting number of columns
print (sheet.ncols)
Output:
3
Code 4: Extract all column names
Python3
# Program extracting all columns
# name in Python
import xlrd
loc = ( "path of file" )
wb = xlrd.open_workbook(loc)
sheet = wb.sheet_by_index( 0 )
# For row 0 and column 0
sheet.cell_value( 0 , 0 )
for i in range (sheet.ncols):
print (sheet.cell_value( 0 , i))
Output:
NAME
SEMESTER
ROLL NO
Code 5: Extract the first column
Python3
# Program extracting first column
import xlrd
loc = ( "path of file" )
wb = xlrd.open_workbook(loc)
sheet = wb.sheet_by_index( 0 )
sheet.cell_value( 0 , 0 )
for i in range (sheet.nrows):
print (sheet.cell_value(i, 0 ))
Output:
NAME
ALEX
CLAY
JUSTIN
Code 6: Extract specific row values
Python3
# Program to extract a particular row value
import xlrd
loc = ( "path of file" )
wb = xlrd.open_workbook(loc)
sheet = wb.sheet_by_index( 0 )
sheet.cell_value( 0 , 0 )
print (sheet.row_values( 1 ))
Output:
['ALEX', 4.0, 2011272.0]
First, your interview preparation enhances your data structure concepts with the Python DS course.