MongoDB is a cross-platform, document-oriented, non-relational (i.e., NoSQL) database program. It is an open-source document database that stores data in the form of key-value pairs.
What is a MongoDB query?
MongoDB queries are used to specify selection filters via query operators while retrieving data from a collection via the db.find() method. We can easily filter documents using query objects. To apply a filter to a collection, we can pass a query specifying the desired document condition as a parameter to this method, which is an optional parameter to the db.find() method.
Query selector:
The following is a list of some of the operators used in MongoDB queries.
operate | grammar | description |
---|---|---|
equal | {“key” : “value”} | Matches a value equal to the specified value. |
Less than | {“key” :{$lt:”value”}} | Matches a value that is less than the specified value. |
Greater than | {“key” :{$gt:”value”}} | Matches a value that is greater than the specified value. |
Less than or equal to | {“key” :{$lte:”value”}} | Matches values that are less than or equal to the specified value. |
Greater than or equal to | {“key” :{$lte:”value”}} | Matches values that are greater than or equal to the specified value. |
Not equal | {“key”:{$ne: “value”}} | Matches all values that are not equal to the specified value. |
Logic with | { “$and”:[{exp1}, {exp2}, …, {expN}] } | The query clause is connected with logical AND, which returns all documents that match the conditions of both clauses. |
Logic or | { “$or”:[{exp1}, { | Concatenating the query clauses with a logical OR will return all documents that match the conditions of either clause. |
Logical non | { “$not”:[{exp1}, {exp2}, …, {expN}] } | Reverses the effect of the query expression and returns documents that don’t match the query expression. |
The database or collection on which we run:
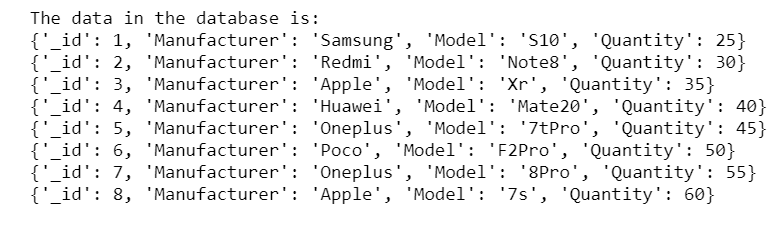
Example 1:
# importing Mongoclient from pymongo
from pymongo import MongoClient
# Making Connection
myclient = MongoClient( "mongodb://localhost:27017/" )
# database
db = myclient[ "mydatabase" ]
# Created or Switched to collection
# names: lsbin
Collection = db[ "lsbin" ]
# Filtering the Quantities greater
# than 40 using query.
cursor = Collection.find({ "Quantity" :{ "$gt" : 40 }})
# Printing the filterd data.
print ( "The data having Quantity greater than 40 is:" )
for record in cursor:
print (record)
# Filtering the Quantities less
# than 40 using query.
cursor = Collection.find({ "Quantity" :{ "$lt" : 40 }})
# Printing the filterd data.
print ( "\nThe data having Quantity less than 40 is:" )
for record in cursor:
print (record)
The output is as follows:
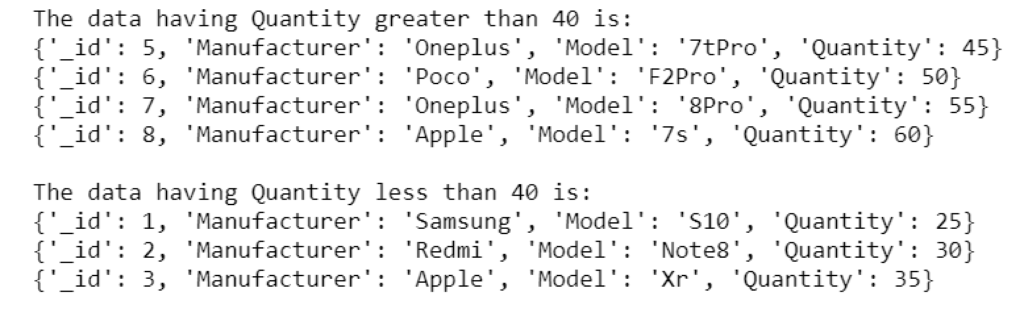
Example 2:
# importing Mongoclient from pymongo
from pymongo import MongoClient
# Making Connection
myclient = MongoClient( "mongodb://localhost:27017/" )
# database
db = myclient[ "mydatabase" ]
# Created or Switched to collection
# names: lsbin
Collection = db[ "lsbin" ]
# Filtering the (Quantities greater than
# 40 AND greater than 40) using AND query.
cursor = Collection.find({ "$and" :[{ "Quantity" :{ "$gt" : 40 }}, { "Quantity" :{ "$gt" : 50 }}]})
# Printing the filterd data.
print ("Quantities greater than 40 AND\
Quantities greater than 40 :")
for record in cursor:
print (record)
# Filtering the (Quantities greater than
# 40 OR greater than 40) using OR query.
cursor = Collection.find({ "$or" :[{ "Quantity" :{ "$gt" : 40 }}, { "Quantity" :{ "$gt" : 50 }}]})
# Printing the filterd data.
print ()
print ("Quantities greater than 40 OR\
Quantities greater than 40 :")
for record in cursor:
print (record)
The output is as follows:
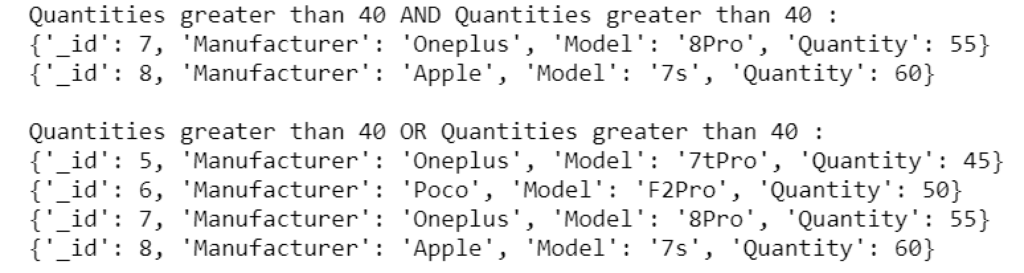
First, your interview preparation enhances your data structure concepts with the Python DS course.