The insertMany() function is used to insert multiple documents into a collection. It accepts a series of documents to be inserted into a collection.
Installation of the Mongoose module:
- You can visit the link to install the Mongoose module. You can use this command to install this package.npm install mongoose
- After installing the Mongoose module, you can use the command to check your version of Mongoose in the command prompt.npm version mongoose
- After that, you can just create a folder and add a file, for example index.js, to run this file you need to run the following command.node index.js
File name :index.js
const mongoose = require( 'mongoose' );
//Database connection
mongoose.connect( 'mongodb://127.0.0.1:27017/lsbin' , {
useNewUrlParser: true , useCreateIndex: true , useUnifiedTopology: true
});
//User model
const User = mongoose.model( 'User' , {
name: { type: String }, age: { type: Number }
});
//Function call
User.insertMany([
{ name: 'Gourav' , age: 20}, { name: 'Kartik' , age: 20}, { name: 'Niharika' , age: 20}
]).then( function (){
console.log( "Data inserted" ) //Success
}). catch ( function (error){
console.log(error) //Failure
});
Steps to run the program:
The project structure will look like this:
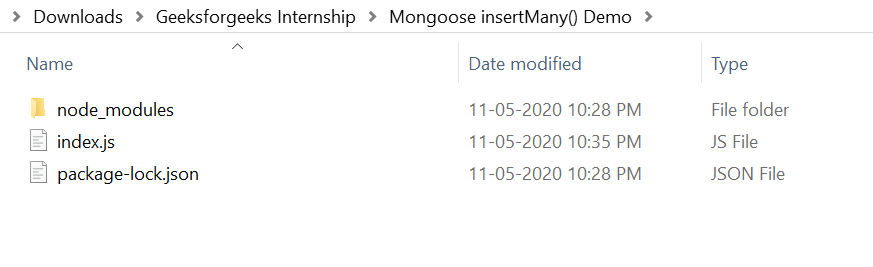
Make sure the mongoose module is installed with the following command: npm install mongoose
Run the index.js file with the following command:
node index.js

After running the above command, you can see that the data has been inserted into the database. You can use any GUI tool or terminal to view the database, just like I did with the Robo3T GUI tool, as follows:
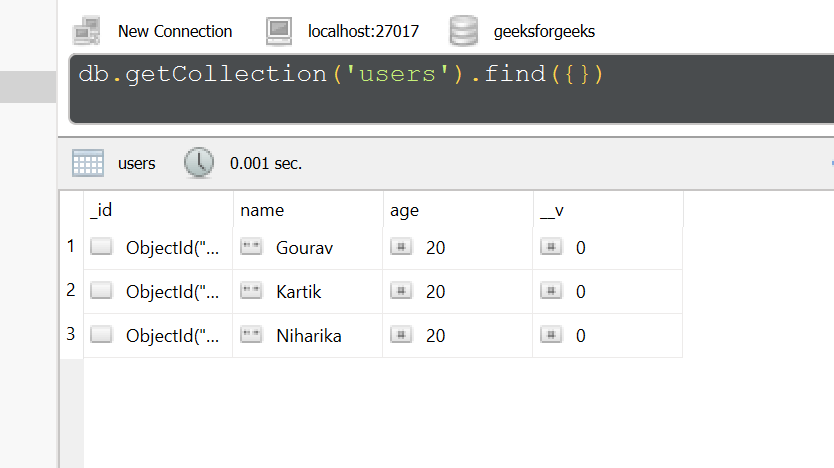
So, this is how to use the mongoose insertMany() function to insert multiple documents into collections in MongoDB and Node.js.