How do I play a sound in Python?
How to play audio using Python? Playing sound using a Python script is a simple task because the language contains many modules that play or record sound using scripts. By using these modules, you can play audio files such as mp3, wav, and other audio file types. You must install the sound module before you can use it in your script. This tutorial will show you how to install different types of Python modules to play sounds and how python can play sounds.
How does python play sound? Use playsound to play sound
This playsound module is the simplest module for playing sound. The module works with both Python 2 and Python 3 and has been tested to only play wav and mp3 files. It contains only a method called playsound() with a parameter for Linux to use to get the audio file name for playback.
Installation:
Run the following pip3 command to install this module in Python 3:
$ pip3 install playsound
Python playback sound example: Use Playsound to play wav and mp3 files
In the following script, first take the wav filename as input, and then play the file using the playsound() method. Next, the mp3 file name will be entered and played through the same method.
#!/usr/bin/env python3
# Import playsound module
from playsound import playsound
# Input an existing wav filename
wavFile = input("Enter a wav filename: ")
# Play the wav file
playsound(wavFile)
# Input an existing mp3 filename
mp3File = input("Enter a mp3 filename: ")
# Play the mp3 file
playsound(mp3File)
Output:
If both files are present, the sound file will start playing.
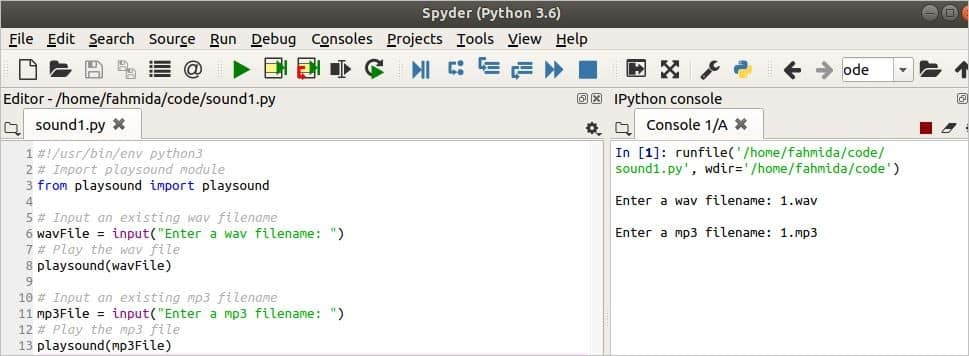
Python’s way to play sound: Play sound with pydub
The pydub module supports Python 2 and Python 3. The pydub module supports different types of audio files. This module can be used to split or attach clips to any audio file. You can also add a simple effect on top of the sound. This module is directly dependent on two other modules, called ffmpeg and libavcodec-extra. These modules must be installed before pydub modules can be installed.
Installation:
Run the following command to install the pydub package for Python:
$ sudo apt-get install ffmpeg libavcodec-extra
$ pip3 install pydub
Example 1: Play local wav and MP3 files
The module uses the form_file() method to play wav files and the form_mp3() method to play MP3 files. Here we use the play() method to play wav and mp3 files:
#!/usr/bin/env python3
from pydub import AudioSegment
from pydub.playback import play
# Input an existing wav filename
wavFile = input("Enter a wav filename: ")
# load the file into pydub
sound = AudioSegment.from_file(wavFile)
print("Playing wav file...")
# play the file
play(sound)
# Input an existing mp3 filename
mp3File = input("Enter a mp3 filename: ")
# load the file into pydub
music = AudioSegment.from_mp3(mp3File)
print("Playing mp3 file...")
# play the file
play(music)
Output:
If both the wav and MP3 filenames are present, a sound will play and the following output will be displayed:
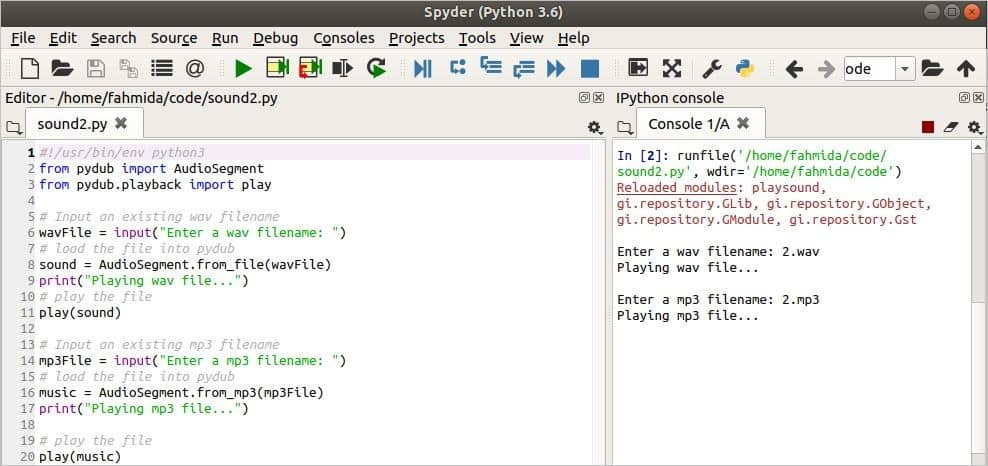
Example 2: Download and play wav and mp3 files from a URL
How to play audio using Python? The following example shows how to play any wav or mp3 file after downloading it from a valid URL location. The urllib module is used in the script to download the sound file. The following is an example of python playing sounds:
#!/usr/bin/env python3
# Import necessary modules
from pydub import AudioSegment
from pydub.playback import play
import urllib
# Set the wav filename
filename = "service-bell.wav"
# Download the wav file from url
print("downloading wav file....")
urllib.request.urlretrieve("http://soundbible.com/grab.php?id=2218&type=wav", filename)
# load the file into pydub
sound = AudioSegment.from_file(filename)
print("Playing wav file...")
# play the file
play(sound)
# Set the mp3 filename
filename = "birds.mp3"
# Download an mp3 file
print("downloading mp3 file....")
urllib.request.urlretrieve("http://soundbible.com/grab.php?id=2207&type=mp3", filename)
# load the file into pydub
birdsound = AudioSegment.from_mp3(filename)
print("Playing mp3 file...")
# Play the result
play(birdsound)
print("Finished.")
Output:
Once the download is complete, the sound file will start to play, and the following output will appear:
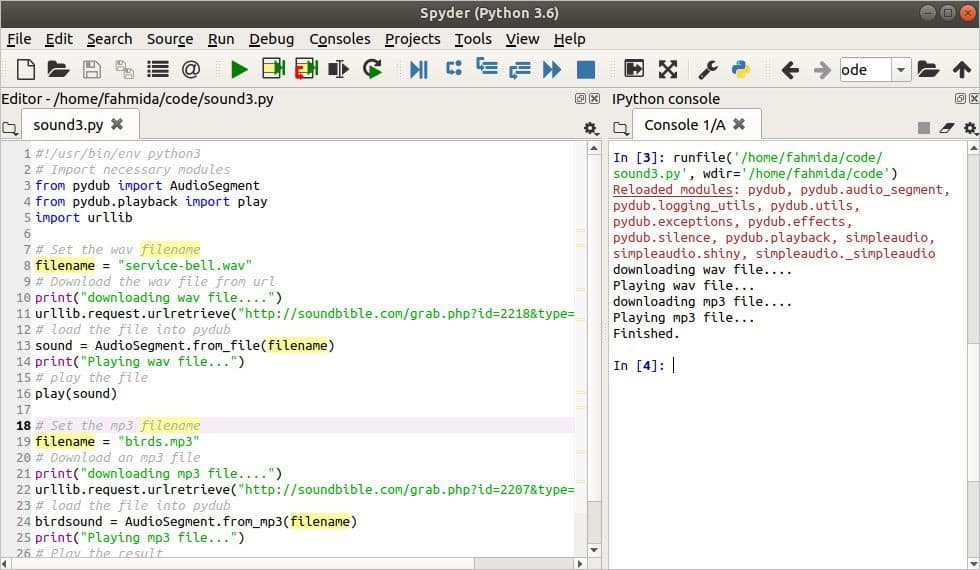
How does python play sound? Use tksnack to play a sound
The tksnack module depends on another module, named Tkinter, to activate the TK script object. The tksnack command can be used after initializing the tk object. You must install the tkinker and tksnack packages for Python 3.
Installation:
$ sudo apt-get install python3-tk
$ sudo apt-get install python3-tksnack
Example: Use tksnack to play a wav file
In the script below, the tkSnack module is initialized with the tkinter object, and the next play() method is used to play the wav file. Here, the blocking parameter specifies that the sound will be played asynchronously.
#!/usr/bin/env python3
# Import necessasry modules
import tkinter
import tkSnack
import os
# Initialize tk object to use tksnack
root = tkinter.Tk()
tkSnack.initializeSnack(root)
#Define tksnack object
sound = tkSnack.Sound()
# Input an existing wav filename
wavFile = input("Enter a wav filename: ")
if os.path.exists(wavFile):
# Read the file
sound.read(wavFile)
# Play sound
sound.play(blocking=1)
else:
# Print the message if the file path does not exist
print ("Wav file does not exist.")
Output:
If the wav file exists, the sound will start playing with the following output:
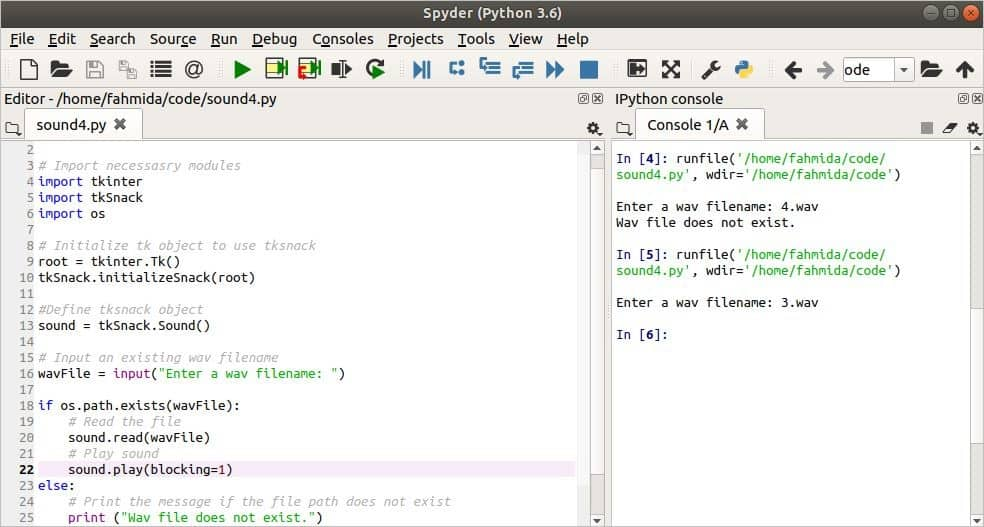
How does python play sound? Use SimpleAudio to play sound
python to play sound method: The simpleaudio module is a package of Python 3 that can play audio sounds. This module is mainly used to play wav files and NumPy arrays. Before you can use this module, you need to install the package. This sound pack is directly dependent on another package called libasound2-dev. You need to install the libasound2-dev package before you can install the simpleaudio package.
Installation:
$ sudo apt-get install libasound2-dev
$ pip3 install simpleaudio
Python playback sound example: Use SimpleAudio to play a WAV file
How to play audio using Python? In the following script, any wav file name will be taken as input. If the file exists, the script will play the sound file; Otherwise, the script displays an error message.
#!/usr/bin/env python3
# Import simpleaudio sound module
import simpleaudio as sa
# Input an existing wav file name
wavFile = input("Enter a wav filename: ")
# Play the sound if the wav file exists
try:
# Define object to play
w_object = sa.WaveObject.from_wave_file(wavFile)
# Define object to control the play
p_object = w_object.play()
print("Sound is playing...")
p_object.wait_done()
print("Finished.")
# Print error message if the file does not exist
except FileNotFoundError:
print("Wav File does not exists")
Output:
A sound file will be played, and the following output will appear after the sound is played:
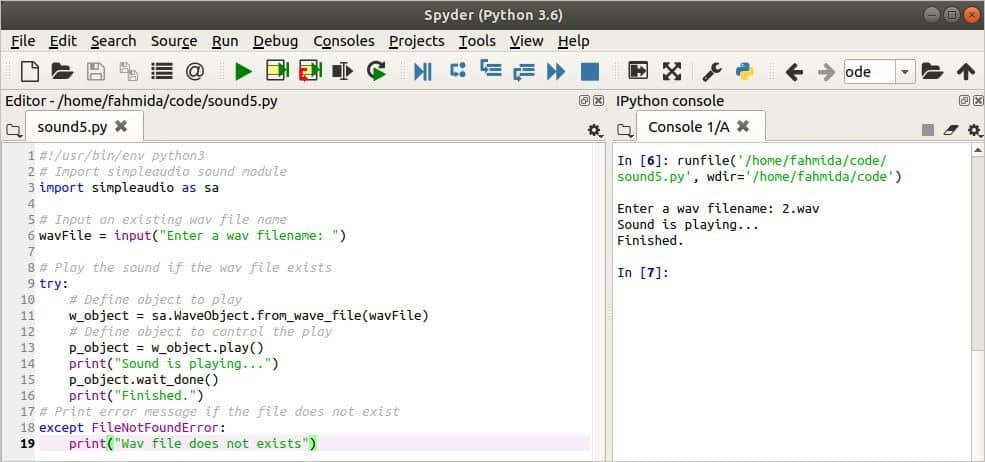
Conclusion
How does python play sound? This tutorial explains the basic purpose of playing sound for four Python modules with several examples. Sound playback tasks are only shown in this tutorial, but you can use Python scripts to record, edit, and different sound-related tasks.