Loops in programming languages are a feature that facilitates the repeated execution of a set of instructions/functions when evaluated true under certain conditions.
Java provides three ways to execute loops. Although all of them provide similar basic functionality, they differ in their syntax and condition checking times.
while loop:
The while loop is a control flow statement that allows code to be executed repeatedly based on a given Boolean condition. A while loop can be thought of as a repetitive if statement.
Syntax:
while (boolean condition)
{
loop statements...
}
Flow chart:

- The While loop starts with a condition check. If the evaluation result is true, the loop body statement is executed, otherwise the first statement after the loop is executed. For this reason, it is also known as an entry control loop
- Once the condition evaluates to true, the statement in the body of the loop is executed. Typically, a statement contains the updated value of the variable to be processed in the next iteration.
- When a condition becomes false, the cycle terminates, marking the end of its life cycle.
// Java program to illustrate while loop
class whileLoopDemo
{
public static void main(String args[])
{
int x = 1 ;
// Exit when x becomes greater than 4
while (x <= 4 )
{
System.out.println( "Value of x:" + x);
// Increment the value of x for
// next iteration
x++;
}
}
}
The output is as follows:
Value of x:1
Value of x:2
Value of x:3
Value of x:4
for loop:
For loops provide a concise way to write loop structures. Unlike while loops, for statements consume initializations, conditions, and increments/decreases in a single line, providing a shorter, easier to debug loop structure.
The syntax is as follows:
for (initialization condition; testing condition;
increment/decrement)
{
statement(s)
}
Flow chart:
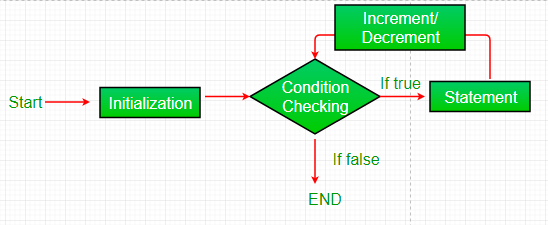
- Initialization Condition: Here, we initialize the variables in use. It marks the beginning of the for cycle. You can use a variable that has already been declared, or you can declare a variable that is only local to the loop.
- Test Condition: The exit condition used to test the loop. It must return a boolean value. This also goes into the control loop, as the condition is checked before the loop statement is executed.
- Statement Execution: Once the condition is evaluated to true, the statement in the body of the loop is executed.
- Increase/Decrease: It is used to update the variable for the next iteration.
- Loop termination: When the condition becomes false, the loop terminates, marking the end of its lifecycle.
// Java program to illustrate for loop.
class forLoopDemo
{
public static void main(String args[])
{
// for loop begins when x=2
// and runs till x <=4
for ( int x = 2 ; x <= 4 ; x++)
System.out.println( "Value of x:" + x);
}
}
The output is as follows:
Value of x:2
Value of x:3
Value of x:4
Enhanced For loop
Java also includes another version of the for loop introduced in Java 5. The enhanced for loop provides an easier way to iterate over the elements of a collection or array. It is inflexible and should only be used when you need to iterate through an element sequentially without knowing the index of the currently processed element.
Also note that when using an enhanced for loop, the object/variable is immutable, i.e., it ensures that the value in the array cannot be modified, so it can be called a read-only loop, where you can’t update the value like any other loop where the value can be modified.
We recommend using this form of for statement whenever possible, rather than using the general form. (ACCORDING TO JAVA DOCUMENTATION)
The syntax is as follows:
for (T element:Collection obj/array)
{
statement(s)
}
Let’s use an example to demonstrate how to use an enhanced for loop to simplify work. Let’s say we have an array of names, and we want to print all the names in that array. Let’s see the difference between these two examples
The enhanced for loop simplifies the following:
// Java program to illustrate enhanced for loop
public class enhancedforloop
{
public static void main(String args[])
{
String array[] = { "Ron" , "Harry" , "Hermoine" };
//enhanced for loop
for (String x:array)
{
System.out.println(x);
}
/* for loop for same function
for (int i = 0; i < array.length; i++)
{
System.out.println(array[i]);
}
*/
}
}
The output is as follows:
Ron
Harry
Hermoine
do-while loop:
The do while loop is similar to the while loop, the only difference is that it checks the condition after the statement is executed, and is therefore an example of exiting the control loop.
The syntax is as follows:
do
{
statements..
}
while (condition);
Flow chart:

- The do while loop starts with the execution of the statement. No conditions were checked for the first time.
- After the statement is executed and the variable value is updated, the condition is checked for truth or falseness. If the evaluation is true, the next iteration of the loop begins.
- When a condition becomes false, the cycle terminates, marking the end of its life cycle.
- It is important to note that a do-while loop will execute its statement at least once before checking any conditions, and is therefore an example of exiting a control loop.
// Java program to illustrate do-while loop
class dowhileloopDemo
{
public static void main(String args[])
{
int x = 21 ;
do
{
// The line will be printed even
// if the condition is false
System.out.println( "Value of x:" + x);
x++;
}
while (x < 20 );
}
}
The output is as follows:
Value of x: 21
Loop traps
Infinite Loop:
One of the most common mistakes when implementing any form of loop is that it may never exit, i.e. the loop runs indefinitely. This happens when a condition fails for some reason.
Example:
//Java program to illustrate various pitfalls.
public class LooppitfallsDemo
{
public static void main(String[] args)
{
// infinite loop because condition is not apt
// condition should have been i>0.
for ( int i = 5 ; i != 0 ; i -= 2 )
{
System.out.println(i);
}
int x = 5 ;
// infinite loop because update statement
// is not provided.
while (x == 5 )
{
System.out.println( "In the loop" );
}
}
}
Another pitfall is that you might add something to your collection object by looping, potentially running out of memory. If you try to execute the following program, after some time, an out-of-memory exception will be thrown.
//Java program for out of memory exception.
import java.util.ArrayList;
public class Integer1
{
public static void main(String[] args)
{
ArrayList<Integer> ar = new ArrayList<>();
for ( int i = 0 ; i < Integer.MAX_VALUE; i++)
{
ar.add(i);
}
}
}
The output is as follows:
Exception in thread "main" java.lang.OutOfMemoryError: Java heap space
at java.util.Arrays.copyOf(Unknown Source)
at java.util.Arrays.copyOf(Unknown Source)
at java.util.ArrayList.grow(Unknown Source)
at java.util.ArrayList.ensureCapacityInternal(Unknown Source)
at java.util.ArrayList.add(Unknown Source)
at article.Integer1.main(Integer1.java:9)
If you find anything incorrect, or would like to share more information about the above topics, please write a comment.