Prerequisite: MongoDB: Introduction
MongoDB is a cross-platform, document-oriented database that handles the concepts of collections and documents. MongoDB provides high speed, high availability, and high scalability.
The next question that comes to people’s minds is “why use MongoDB?”
Reasons to choose MongoDB:
- It supports hierarchical data structures (see docs for details)
- It supports associative arrays, such as Dictionary in Python.
- Built-in Python driver to connect python applications to databases. Example – PyMongo
- It’s designed for big data.
- MongoDB is very easy to deploy.
MongoDB and RDBMS
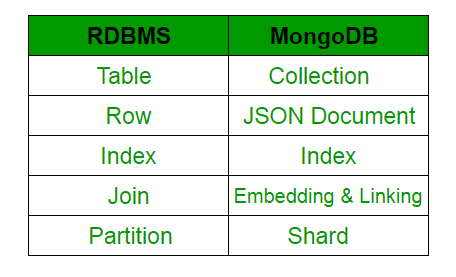
MongoDB and PyMongo Installation Guide
Start MongoDB from the command prompt with the following command:
Method 1:
mongod
or
Method 2:
net start MongoDB
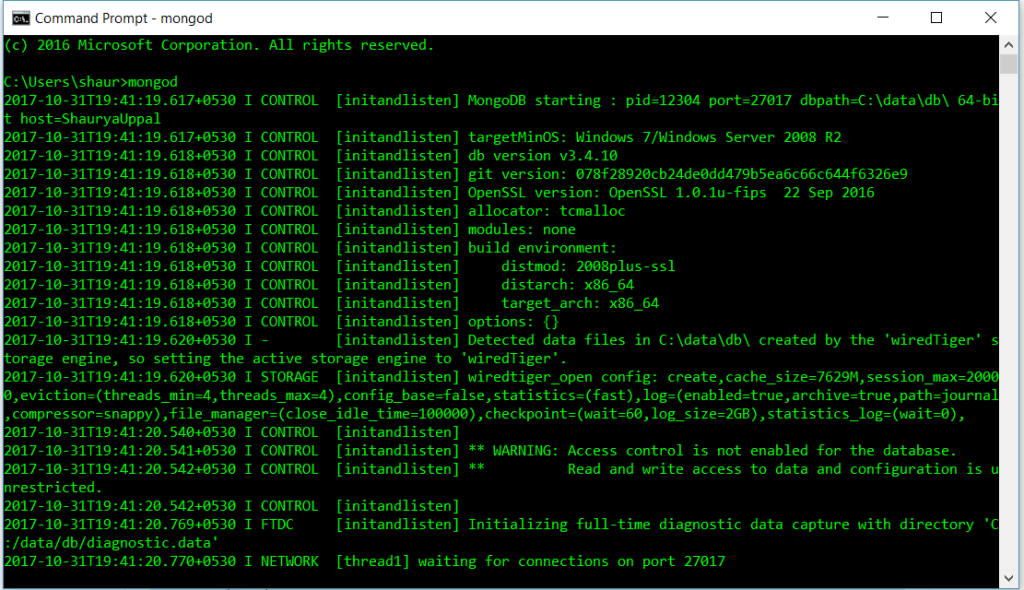
See Setting the port number to 27017 by default (last row in the image above).
Python has native libraries for MongoDB. The name of the available library is “PyMongo”. To import this file, execute the following command:
from pymongo import MongoClient
Create a connection:
The first step after importing the module is to create a MongoClient.
from pymongo import MongoClient
client = MongoClient()
After that, connect to the default host and port. The connection to the host and port is explicitly complete. The following command is used to connect to MongoClient on a local host running on port 27017.
client = MongoClient(‘host’, port_number)
example: - client = MongoClient(‘localhost’, 27017 )
This can also be done using the following command:
client = MongoClient("mongodb: //localhost: 27017 /")
To access database objects:
To create a database or switch to an existing database, we use:
Method 1: Dictionary style
mydatabase = client[‘name_of_the_database’]
Method 2:
mydatabase = client.name_of_the_database
If there is no database previously created with that name, MongoDB will implicitly create a database for the user.
Note: Database-populated names cannot use any of the dashes (-) in them. Names like my-table will throw an error. Therefore, the use of underscores in names is allowed.
Access the collection:
A collection is equivalent to a table in an RDBMS. We access collections in PyMongo in the same way that we access tables in RDBMS. To access the table, say the table name of the database “myTable”, say “mydatabase”.
Method 1:
mycollection = mydatabase[‘myTable’]
Method 2:
mycollection = mydatabase.myTable
> MongoDB stores the database in the form of a dictionary, as follows:
record = {
title: 'MongoDB and Python', description: 'MongoDB is no SQL database', tags: ['mongodb', 'database', 'NoSQL'], viewers: 104
}
“_id” is a special key that is automatically added if the programmer forgets to add it explicitly. _id is a 12-byte hexadecimal number that ensures the uniqueness of each inserted document.

To insert data into a collection:
Method used:
insert_one() or insert_many()
We usually use insert_one() method documentation in our collections. Let’s say we want to enter data named a record into a “myTable” of “mydatabase”.
rec = myTable.insert_one(record)
When it needs to be executed, the whole code looks like this.
# importing module
from pymongo import MongoClient
# creation of MongoClient
client = MongoClient()
# Connect with the portnumber and host
client = MongoClient("mongodb: //localhost: 27017 /")
# Access database
mydatabase = client[‘name_of_the_database’]
# Access collection of the database
mycollection = mydatabase[‘myTable’]
# dictionary to be added in the database
rec = {
title: 'MongoDB and Python' , description: 'MongoDB is no SQL database' , tags: [ 'mongodb' , 'database' , 'NoSQL' ], viewers: 104
}
# inserting the data in the database
rec = mydatabase.myTable.insert(record)
Query in MongoDB:
There are certain query functions that can be used to archive data in a database. The two most commonly used features are:
find()
find() is used to get multiple single documents as query results.
for i in mydatabase.myTable.find({title: 'MongoDB and Python' })
print (i)
This will output all documents titled “MongoDB and Python” in myTable, mydatabase.
count()
count() is used to get the number of documents whose names are passed in via the parameter.
print (mydatabase.myTable.count({title: 'MongoDB and Python' }))
This will output the number of documents titled “MongoDB and Python” in myTable’s myTable.
These two query functions can be summed to get the best filtered results, as shown below.
print (mydatabase.myTable.find({title: 'MongoDB and Python' }).count())
To print all documents/entries inside “myTable” of database “mydatabase”:
Use the following code:
from pymongo import MongoClient
try :
conn = MongoClient()
print ( "Connected successfully!!!" )
except :
print ( "Could not connect to MongoDB" )
# database name: mydatabase
db = conn.mydatabase
# Created or Switched to collection names: myTable
collection = db.myTable
# To find() all the entries inside collection name 'myTable'
cursor = collection.find()
for record in cursor:
print (record)
This article was written by Rishabh Bansal and Shaurya Uppal.
If you find anything incorrect, or would like to share more information about the above topics, please write a comment.
First, your interview preparation enhances your data structure concepts with the Python DS course.